Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial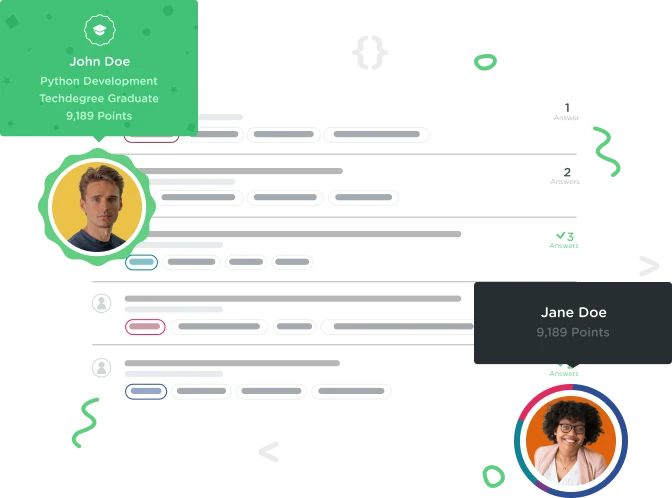
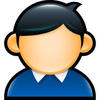
Daniel Hildreth
16,170 PointsNeed Clarification and Explanation On This C# Code
So I have the following code from the Delegates video in the Querying with Linq course. I need some help on understanding some bits on it. The first thing that I need help with is this line:
Console.WriteLine(string.Format("Hello, {0}", name));
I understand passing in the text and the name property. However, what is the {0} for? How is that used to get the input name later in the code? The last bit I need help on is actually basic stuff, but have forgotten what it means:
SayGreeting sayGreeting = new SayGreeting(SayHello);
I know that the sayGreeting part is basically naming the method so we can use it later on. The new SayGreeting(SayHello) is saying we want to create a new SayGreeting method and pass in the SayHello method. The part I'm a little confused on is the first part. We write SayGreeting before it all, and I have forgotten what part of the code this is and why we need it. Hopefully my question makes sense, and that someone can help re-explain this to me.
using System;
namespace FunctionalProgramming
{
class Program
{
delegate void SayGreeting(string name);
public static void SayHello(string name)
{
Console.WriteLine(string.Format("Hello, {0}", name));
}
public static void SayGoodbye(string name)
{
Console.WriteLine(string.Format("Later, {0}", name));
}
static void Main(string[] args)
{
SayGreeting sayGreeting = new SayGreeting(SayHello);
Console.WriteLine("What's your name?");
string input = Console.ReadLine();
sayGreeting(input);
Console.ReadLine();
sayGreeting = new SayGreeting(SayGoodbye);
sayGreeting(input);
}
}
}
1 Answer
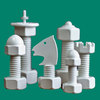
Steven Parker
231,222 PointsPerhaps this will clarify things a bit:
In this code: "string.Format("Hello, {0}", name)
", the "{0}" in the string is a placeholder that shows the Format method where you want the first argument (name) to be inserted. So if name contains "Daniel" here, the resulting string produced by Format will be "Hello, Daniel".
Then for this code: "SayGreeting sayGreeting = new SayGreeting(SayHello)
", remember that SayGreeting is a method delegate. That means it represents some other method. So here, sayGreeting (little "s") is being declared as a SayGreeting type, which means it will be a method that requires a string but returns nothing (void). Then it is assigned a new SayGreeting(SayHello) which means when sayGreeting is called, it will perform as if SayHello was called instead.
Does that help?