Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial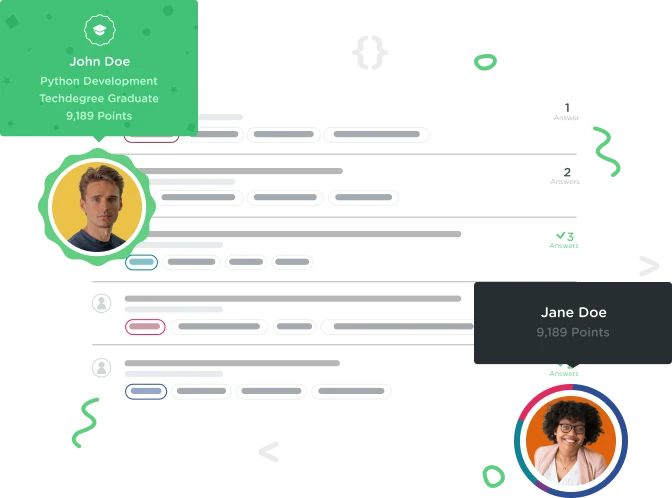

Yousif Sayer
7,073 PointsNeed help in Exception challenge I don't know where is the error
In this challenge is asking to throw an exception. I tried with try catch blocks but it seems there is an error in the way I did.
Can anyone help.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
try{
lapsDriven += laps;
barCount -= laps;
}catch(IllegalArgumentException e){
System.out.print("There is no enogh bars!");
}
}
}
2 Answers

andren
28,558 PointsTry/Catch block are used to catch exceptions from code that throws them, it is not used to actually throw exceptions.
Throwing an exception is done with code along these lines:
throw new IllegalArgumentException();
But in addition to throwing the exception you have to yourself implement a test to see if the exception should be thrown. Basically you need to write an if statement that checks if there is enough battery to complete the lap.
I recommend you try to solve the challenge again now that you have a better idea about what you have to do, but if you still can't solve it then I have posted the solution for you here.
Edit: Changed the linked solution a bit to address a logic issue.
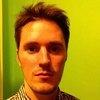
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsYour code right now doesn't throw an error if someone tries to drive more laps then they have bars left. We'll end up with a negative number of bars, which is a logic problem, but it doesn't throw an error. You need to force it to throw an error if it meets the condition:
public void drive(int laps) {
if (laps >= barCount) {
throw new IllegalArgumentException("There is not enough bars!");
} else {
lapsDriven += laps;
barCount -= laps;
}
}

andren
28,558 PointsThere is a slight logic error in your code, the condition should be "laps > barCount". With your condition it would throw an error if 1 lap was requested while there was 1 bar of battery, which should work.
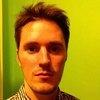
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsCool, thanks for pointing that out.