Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial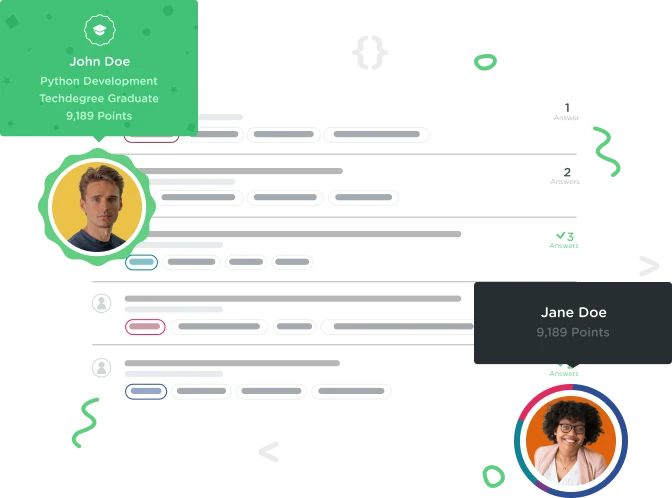

kishan becks
30 PointsNeed help with Basic C++ programming
this is the problem :
classify-by-age which accepts one value, a non-negative integer, and display the message INVALID if the value is less than 2, display the message TRY AGAIN if the value is less than or equal to 12, display the message FAILED if the value is less than or equal to 18, display the message CHECK THE RESULT if the value is less than 60, and display the message REPEAT in any other case.
tq .
this is what i did so far .
#include <iostream>
using namespace std;
int main()
{
int age = 60;
int age2;
cout << "Please Enter Age\n";
cin >> age;
if ( age >19 && age <= 60) {
cout << "CHECK THE RESULTS";
}
else if(age >12 && age <= 18 ) {
cout << "FAILED";
}
else if(age >=2 && age <= 12 ) {
cout << "TRY AGAIN";
}
else if(age < 2)
{
cout << "INVALID";
}
else if(age > 60)
{
cout << "REPEAT";
}
cin >> age2;
return 0;
}
2 Answers
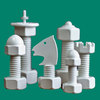
Steven Parker
231,007 Pointsclassify-by-age which accepts one value, a non-negative integer, and display the message INVALID if the value is less than 2, display the message TRY AGAIN if the value is less than or equal to 12, display the message FAILED if the value is less than or equal to 18, display the message CHECK THE RESULT if the value is less than 60, and display the message REPEAT in any other case.
It seems like part of the instructions are cut off at the beginning. What comes before "classify-by-age which accepts one value ..."? Could it be that it was asking for a function by that name?
Otherwise, there appear to be some basic logic issues:
What the instructions ask for: | What this code will do: |
---|---|
display INVALID if the value is less than 2 | |
display TRY AGAIN if the value is less than or equal to 12 | |
display FAILED if the value is less than or equal to 18 | |
display CHECK THE RESULT if the value is less than 60 | display "CHECK THE RESULTS" (plural) |
display REPEAT in any other case | display "CHECK THE RESULTS" if value = 60 |
(not asked for) | wait on another input |
The best way to resolve the logic issues might be to perform the tests in the same order as they are described in the instructions. Then you only need to test against one value at a time.