Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial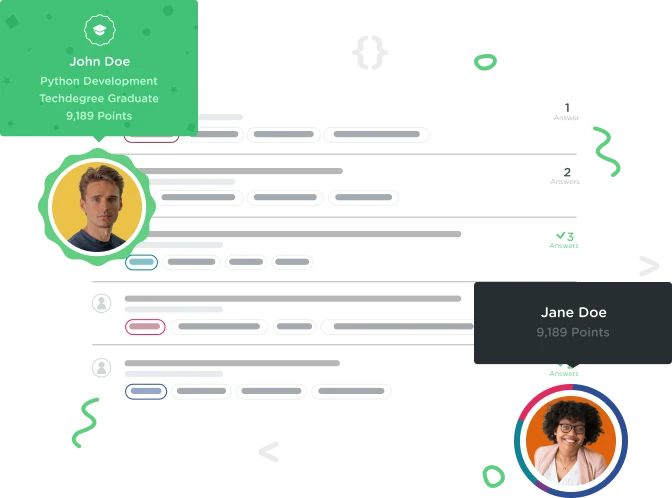

matthew walter
6,618 PointsNext, you need to get all of the plants in the database so they can be displayed on the homepage. Inside of the index fu
Next, you need to get all of the plants in the database so they can be displayed on the homepage. Inside of the index function, gather all of the plants with .query.all() and pass them into the template. Use plants= inside of render_template() and set it equal to the plants
from models import db, Plant, app
from flask import render_template, url_for, request, redirect
@app.route('/')
def index()
render_template(plants=Plant.query.all())
return render_template('index.html', plants=Plant.query.all())
@app.route('/form', methods=['GET', 'POST'])
def form():
if request.form:
new_plant = Plant(plant_type=request.form['type'],
plant_status=request.form['status'])
db.add(new_plant)
db.commit()
return redirect(url_for('index'))
return render_template('form.html',)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///plants.db'
db = SQLAlchemy(app)
class Plant(db.Model):
id = db.Column(db.Integer, primary_key=True)
plant_type = db.Column(db.String())
plant_status = db.Column(db.String())
{% extends 'layout.html' %}
{% block content %}
<form action="{{ url_form('form') }}" method="POST">
<label for="type">Plant Type:</label>
<input type="text" name="type" id="type" placeholder="Plant type">
<p>Plant Status:</p>
<label for="good">GOOD</label>
<input type="radio" name="status" id="good">
<label for="ok">OK</label>
<input type="radio" name="status" id="ok">
<label for="bad">BAD</label>
<input type="radio" name="status" id="bad">
</form>
{% endblock %}
{% extends 'layout.html' %}
{% block content %}
<h1>Our Plants:</h1>
<div>
<h2>Plant Type</h2>
<p>Plant Status</p>
</div>
{% endblock %}
1 Answer
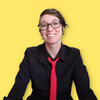
Mel Rumsey
Treehouse ModeratorHi matthew walter !
You are calling render template twice. We just want the return statement to call the render template method. The plants = Plant.query.all()
that you have on line 7 is correct, but it doesn't need to be wrapped in the render template. Then in the render_template on line 8, we can set plants=plants
. We won't need to query the database again since we are using the variable that is storing all of the plants.
Hopefully this helps! Let me know if you have any questions.