Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial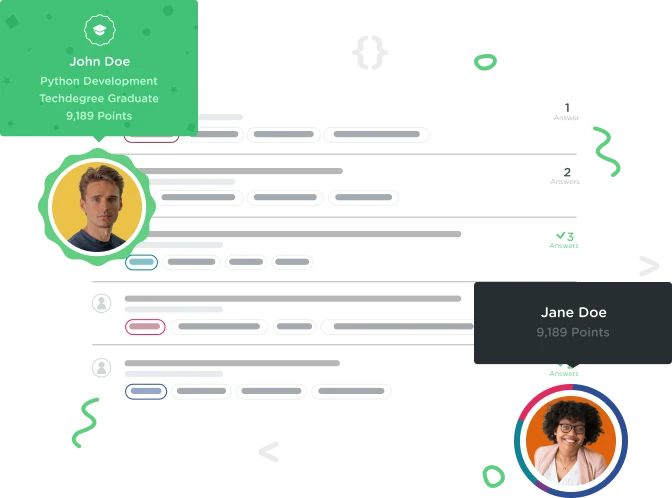
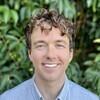
Asher Orr
Python Development Techdegree Graduate 9,408 PointsNot receiving "{} is neither a fizzy or buzzy number" when entering numbers not divisible by 3 or 5.
Hi everyone! I created a function to calculate whether a number is a FizzBuzz, a Fizz, a Buzz, or neither fizzy or buzzy.
Here's my code:
def fizz_buzz_calculator(number):
if number % 3 == 0 and number % 5 == 0:
print("{} is a FizzBuzz number.".format(number))
elif number % 3==0:
print("{} is a Fizz number.".format(number))
elif number % 5==0:
print("{} is a Buzz number.".format(number))
elif number % 3 == 1 and number % 5 == 1:
print("{} is neither a fizzy or a buzzy number.".format(number))
name = input("Please enter your name: ")
try:
number = int(input("Please enter a number: "))
except ValueError:
print("Please enter only a whole number.")
else:
print("Hi, {}!".format(name))
print("You entered the number {}.".format(number))
fizz_buzz_calculator(number)
If I understand correctly, my function is saying:
- if the number is evenly divisible by 3 and 5, print "is a Fizzbuzz number."
- if that's not true, try dividing the number by 3. If it's evenly divisible by 3, print "is a fizz number."
- if that's not true, try dividing the number by 5. If it's evenly divisible by 5, print "is a buzz number."
- If none of the above arguments are true, try seeing if the number is not evenly divisible by 3 and 5. If that's the case, print "{} is neither a fizzy or a buzzy number."
When I pass numbers like 15, 5, and 6, my code works. But when I pass a number that isn't evenly divisible by either 3 or 5, like the number 8, my function doesn't run.
The last thing I see in the console is "You entered the number {}."
Can anyone help me here? Thanks so much for reading!
EDIT:
Hello again! Shortly after posting the question above, I updated my code:
def fizz_buzz_calculator(number):
if number % 3 == 0 and number % 5 == 0:
print("{} is a FizzBuzz number.".format(number))
elif number % 3==0:
print("{} is a Fizz number.".format(number))
elif number % 5==0:
print("{} is a Buzz number.".format(number))
else:
print("{} is neither a fizzy or a buzzy number.".format(number))
name = input("Please enter your name: ")
try:
number = int(input("Please enter a number: "))
except ValueError:
print("Please enter only a whole number.")
else:
print("Hi, {}!".format(name))
print("You entered the number {}.".format(number))
fizz_buzz_calculator(number)
It's working now, but I'm confused as to why the original function did not work. I would appreciate it if someone could explain to me what was happening! Thank you!
2 Answers

jb30
44,806 Pointsnumber % 3 == 1 and number % 5 == 1
will be True
when number
is 1
more than a multiple of 15
, and False
otherwise.
There are three possibilities for number % 3
: 0
, 1
, and 2
. There are five possibilities for number % 5
: 0
, 1
, 2
, 3
, and 4
. The previous elif
statements dealt with the cases of number % 3 == 0
and number % 5 == 0
, so in the final elif
statement, number % 3
will be 1
or 2
and number % 5
will be 1
, 2
, 3
, or 4
.
When number
is 8
, 8 % 3
is 2
, which is not equal to 1
, so the condition is False
and nothing gets printed.
When number
is 7
, 7 % 3
is 1
, which is equal to 1
, but 7 % 5
is equal to 2
, which is not equal to 1
. True and False
becomes False
, so nothing gets printed.
When number
is 16
, 16 % 3
is 1
and 16 % 5
is 1
. 1 == 1 and 1 == 1
is True
, so the original code will print 16 is neither a fizzy or a buzzy number.
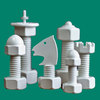
Steven Parker
231,007 PointsYour final test in the first example is elif number % 3 == 1 and number % 5 == 1
and neither of those conditions would be true for the number 8. It would work only for numbers like 1, 16, 31, 46 … etc., and that's why you didn't see the final message.
The important thing is that you realized that you needed just a plain "else" to handle all numbers not previously identified.