Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial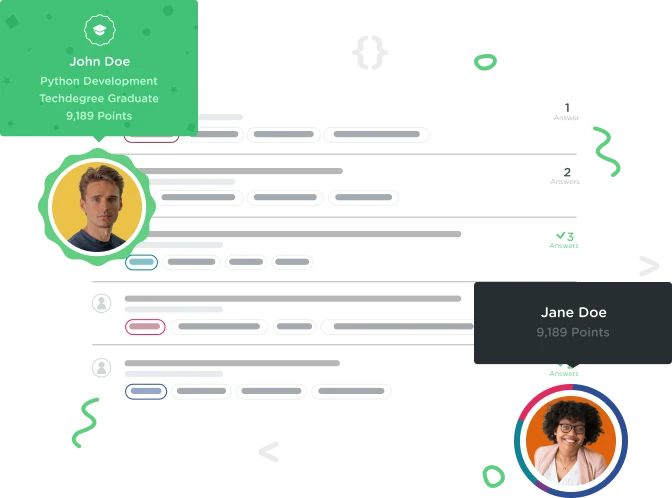

Godfrey Adiges
2,146 PointsNow replace your name with the firstName variable using the string formatter.
How would I complete this exercise?
3 Answers

Ken Alger
Treehouse TeacherGodfrey;
Let's walk through this entire challenge as your particular question comes up quite frequently.
Task 1
Define a string variable named firstName
that stores your name. Set the value to your name.
It sounds like you have gotten through this task, but here is the code:
// Course: Java Basics
// Module: Getting Started with Java
// Challenge: Strings, Variables, and Formatting
// Task: 1 of 3
String firstName = "Godfrey";
Task 2
Call the print function on the console object and make it print out "<YOUR NAME>
can code in Java!"
Again, it sounds like you got this far as well.
// Course: Java Basics
// Module: Getting Started with Java
// Challenge: Strings, Variables, and Formatting
// Task: 2 of 3
String firstName = "Godfrey";
console.printf("Godfrey can code in Java!");
Task 3
Now replace your name with the firstName
variable using the string formatter.
This is where folks seem to be having an issue. In the video he covers the Java string formatter %s
in better detail but think of it as a substitution of sorts. When you put the %s
in the string Java says "Okay, I'll substitute that with whatever variable the programmer tells me to." In our case we want it to be the firstName
string variable. Again, the video shows the syntax of how to do that, but after we close our printf
quote we put a comma and then type our variable name. So we would do printf("%s can code in Java!", firstName)
. That would turn our challenge code into:
// Course: Java Basics
// Module: Getting Started with Java
// Challenge: Strings, Variables, and Formatting
// Task: 3 of 3
String firstName = "Godfrey";
console.printf("%s can code in Java!", firstName);
So now, in this example, when the code runs Java will insert whatever value has been assigned to firstName
(Godfrey in this case) in place of the string formatter %s
.
I hope that makes some sense.
Ken
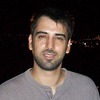
Michalis Efstathiou
11,180 Pointsconsole.printf(firstName + " can code in Java!");

Ken Alger
Treehouse TeacherMichalis;
The code is asking to use "the string formatter" not to concatenate.
Ken

Alwin Joy
1,143 Pointsthanks brother
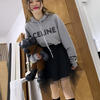
Kevin Narain
11,379 PointsIf you are using an IDE this code will work :)
package com.company;
import java.io.Console;
import java.util.Scanner;
public class Main {
// Main method
public static void main(String[] args) {
Console console = System.console(); // Console object.
// Welcome to the Introductions program! Your code goes below here.
Scanner firstNameInput = new Scanner(System.in); // Create a scanner object for input.
System.out.println("What is your first name?"); // Ask user to enter first name.
String firstName = firstNameInput.nextLine(); // Receive the user input.
System.out.println("I'm " + firstName + " and I'm hot"); // Output what is received.
System.out.println(firstName + " is learning Java."); // Output what is received.
}
}
Godfrey Adiges
2,146 PointsGodfrey Adiges
2,146 PointsOh ok now I got it. Thanks
brennanroy
2,394 Pointsbrennanroy
2,394 PointsHi Ken:
I have followed these instructions numerous times and can't seem to get past Challenge Task 3 of 3 in this Java Basics Course.
My final answer looks to be in exact order that you have it.
String firstName = "Ben"; console.printf ("%s can code in Java!", firstName);
The error message = Please make sure you leave your printf statement intact.