Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial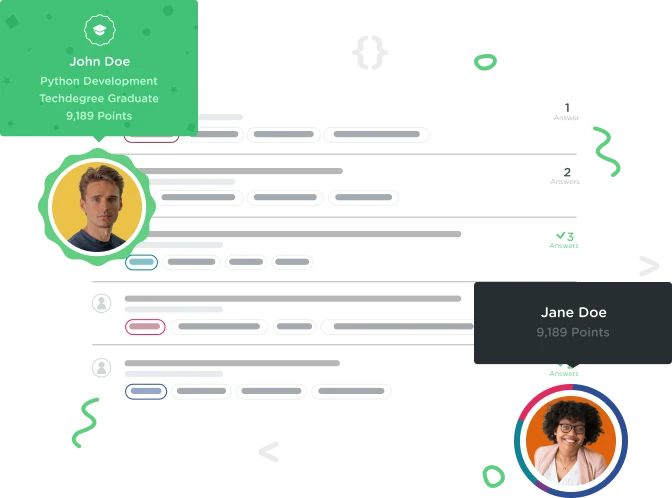

danielchristie
21,505 PointsOn the Basic C# Final. My Code works outside of the test environment but receives an error inside...
I am to print "Yay!" a certain number of times defined by the user. I am also to validate the user's entry, ensuring that the user's entry is not a decimal value nor letter. Whenever I run this code on my own outside of the Final test environment, I receive no error and my code words perfectly as requested however, when I submit my code in the final, it says It sees an error. I am confused and not sure what it wants...
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
bool persist = true;
int cycles = 0;
while (persist)
{
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
var reply = Console.ReadLine();
try
{
cycles = int.Parse(reply);
persist = false;
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
for (int count = 0; count < cycles; count++)
{
Console.WriteLine("Yay!");
}
}
}
}
4 Answers
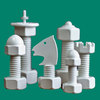
Steven Parker
231,248 PointsIt looks like you have discovered a bug in the "check work" mechanism. You should submit a report and get yourself an "exterminator" badge!
In the meantime, while your loop is a more elegant solution, the stated objective doesn't require it. Just eliminate the loop and it will pass the test:
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int cycles = 0;
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
var reply = Console.ReadLine();
try
{
cycles = int.Parse(reply);
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
return;
}
for (int count = 0; count < cycles; count++)
{
Console.WriteLine("Yay!");
}
}
}
}

danielchristie
21,505 PointsThank you very much for your help folks, appreciated.

Greg McIntyre
4,208 PointsI tried it differently. Mind telling me why this simple code won't work? Im new using for loops, and the error says "the name i does not exist in this context.." ?
static void Main() { Console.Write("Enter the number of times to print \"Yay!\": "); string entry = Console.ReadLine(); int numberOfYays = int.Parse(entry);
for (i = 1; i <= numberOfYays; i++){
Console.WriteLine("Yay!");
break;
}
}
}
}

Seth Kroger
56,413 PointsThe break statement will break out of the loop in the first go-around, so "Yay!" will only be printed once.

Seth Kroger
56,413 PointsThen you need to put a "var" or "int" in front of it to declare it.

Greg McIntyre
4,208 PointsThanks. I took the break out, but it still says " i doesn't exist in this context".

Greg McIntyre
4,208 PointsI figured it out. Idk how yours above worked now, bc you never declared count before using it in the for loop???
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsWhen you submit the code does it give you a compile or runtime error? Or does it say something like "Bummer, not what I expected"? It the latter, what are the precise instructions of the code challenge?