Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial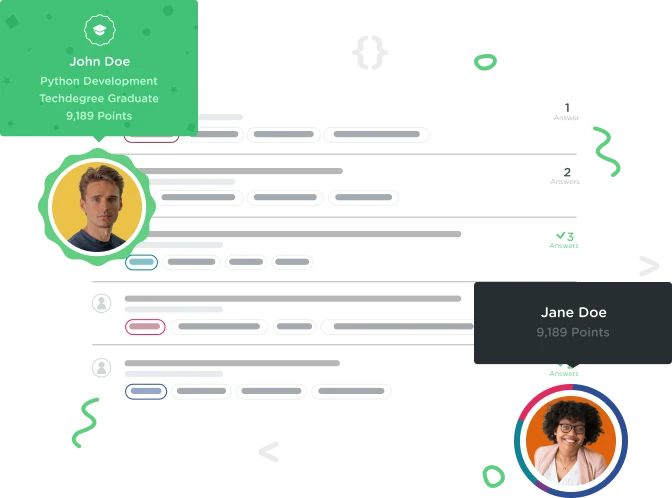
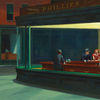
Anthony Grodowski
4,902 Pointspeewee.InterfaceError: Error binding parameter 0 - probably unsupported type.
To be honest I don't even know what that error means hah! Can someone find out why is it happening? I can't find any typos.
#!/usr/bin/env python3
# chmod +x diary.py
# that shabang line tells that its python3. It's equal to python3 diary.py. If I'm on windows, it isnt neccessary
from collections import OrderedDict
import datetime
import sys
from peewee import *
db = SqliteDatabase('diary.db')
class Entry(Model):
# content
content = TextField() # it allows us to put as much text as we want
# timestamp
timestamp = DateTimeField(default = datetime.datetime.now) # Default is seen by python as a funcion and it will call it whenever the entry is created and that way we get the time now. Otherwise it the time would be when we ran the script
class Meta:
database = db
def initialize():
"""Create the database and the table if they don't exist"""
db.connect()
db.create_tables([Entry], safe=True)
def menu_loop():
"""show menu"""
choice = None
while choice != 'q':
print('Enter q to quit')
for key, value in menu.items():
print('{}) {}'.format(key, value.__doc__)) # __doc__ - a magic variable that holds the docstring of a function, method, or class
choice = input('Action: ').lower().strip()
if choice in menu:
menu[choice]()
def add_entry():
"""add an entry"""
print("Enter your entry. Press ctrl+d when finished.")
# now we need to actualy capture their input
data = sys.stdin.read().strip() # stdin is like a keyword, read brings in all the data and strip gets rid of any of the white space on either side
# now we gotta make sure that we actually got smth from them
# sys.stdin - a Python object that represents the standard input stream. In most cases, this will be the keyboard
if data:
if input('Save entry? [y/n] ').lower() != 'n':
Entry.create(content=data)
print("Saved succesfully!")
def view_entries():
"""view previous entries"""
entries = Entry.select().order_by(Entry.timestamp.desc)
for entry in entries:
timestamp = entry.timestamp.strftime('%A %B %d, %Y %I:%M%p') # A weekday name, B month name, d number, Y year, I hour, M minute, p am or pm
print(timestamp)
print('='*len(timestamp))
print(entry.content)
print('n) next entry')
print('q) return to main menu')
next_action = input('Action: [nq] ').lower().strip()
if next_action == 'q':
break
def delete_entry(entry):
"""delete an entry"""
menu = OrderedDict([ # the only difference is that it remembers the order that the keys went in and they always come back in the same order
('a', add_entry),
('v', view_entries)
])
if __name__ == '__main__':
initialize()
menu_loop()
Error:
a) add an entry
v) view previous entries
Action: v
Traceback (most recent call last):
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2780, in execute_sql
cursor.execute(sql, params or ())
sqlite3.InterfaceError: Error binding parameter 0 - probably unsupported type.
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "diary.py", line 83, in <module>
menu_loop()
File "diary.py", line 40, in menu_loop
menu[choice]()
File "diary.py", line 61, in view_entries
for entry in entries:
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2483, in __iter__
return iter(self.execute())
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2476, in execute
self._qr = ResultWrapper(model_class, self._execute(), query_meta)
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2172, in _execute
return self.database.execute_sql(sql, params, self.require_commit)
return self.database.execute_sql(sql, params, self.require_commit)
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2788, in execute_sql
self.commit()
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2657, in __exit__
reraise(new_type, new_type(*exc_value.args), traceback)
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 115, in reraise
raise value.with_traceback(tb)
File "/usr/local/lib/python3.6/site-packages/peewee.py", line 2780, in execute_sql
cursor.execute(sql, params or ())
peewee.InterfaceError: Error binding parameter 0 - probably unsupported type.
1 Answer
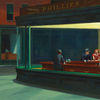
Anthony Grodowski
4,902 PointsAlright, i forgot parens in entries = Entry.select().order_by(Entry.timestamp.desc())