Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial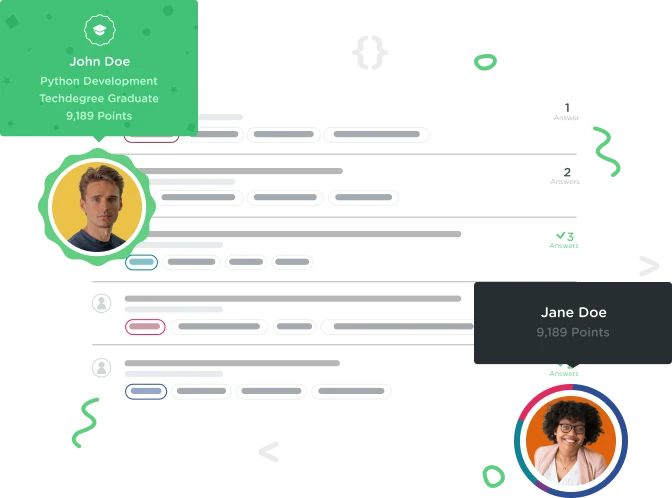

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsPractice Basic Math Calculations in Python
Questions:
• 1.Let f(w) = 2w^3 - 5. What is f(4)? Answer: f(4) = 2*4^3 - 5 = 2*64 - 5 = 128 - 5 = 123.
• 2. Let f(w) = 2w^3 - 5. Let z = 2. What is f(z)? Answer: f(2)=2(2)^3-5 = 32-5 =27
• 3.Let g(x) = cos(x/2) - 3x. Let f(x) = 2x + 5. What is g(f(x))? Answer: 𝑓(𝑥)=2𝑥+5f(x)=2x+5 𝑔(𝑥)=cos(𝑥2)−3𝑥g(x)=cos(x2)−3x 𝑔(𝑓(𝑥))=cos(𝑓(𝑥)2)−3𝑓(𝑥)g(f(x))=cos(f(x)2)−3f(x) 𝑔(𝑓(𝑥))=cos(2𝑥+52)−3(2𝑥+5)g(f(x))=cos(2x+52)−3(2x+5) 𝑔(𝑓(𝑥))=𝐜𝐨𝐬(𝑥+52)−6𝑥−15
• 4.Let f(x) = tan(x) - 2/x. Let g(x) = x^2 + 8. What is f(x)*g(y)?
Answer: f(x)g(y) =(tanx-2/x)(y²+8) =y²tanx+8tanx-2y²/x-16/x
• 5.What is the derivative of x^2 - x + 3 at the point x = 5? Answer: y=x^2-x+3 = dy/dx= 2x-1 = dy/dx at ( x=5 ) is =2×5–1. = 9
• 6.What is the value of x where x^2 - x + 3 is a minimum? Answer: d/dx(x^2-x+3) = 2x-1 = 2x-1 = 0 => x = 1/2
• 7.In Python, what is returned when evaluating [n for n in range(10) if n % 2]? Answer: >>> [n for n in range(10) if n % 2] [1, 3, 5, 7, 9]
• 8.In Python, what kind of error is returned by the following code? (e.g. NameError, ValueError, IOError, etc.) def my_func(n1, n2): return n1 + n2 my_func(1, 2, 3)
Answer: def my_func (n1, n2):
return n1+n2
my_func (1, 2, 3)
def my_func2 (n1, n2):
return n1+n2
my_func2 (1, 2, 3]
• 9.What is returned by datetime(1970, 1, 1).strftime('%Y-%d-%B') in Python? Answer:
date time(1970, 1, 1).strftime('%Y-%d-%B') ^ SyntaxError: invalid syntax
or
---> 10 datetime(1970, 1, 1).strftime('%Y-%d-%B') TypeError: 'module' object is not callable
Any Hints, comments and suggestions are very much welcome, I have a couple of questions I tried to answer please correct me if I am wrong, thank you in advance for your help.

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsHello Mr.Freeman apologies for the inconvenience but I have not tried anything yet because I don't know what to do that is why at the end of the post I was asking for hints,comments and suggestions not the actual answer, I hope that clears up the misunderstanding. Have a good one :)
1 Answer
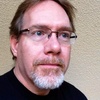
Chris Freeman
Treehouse Moderator 68,426 PointsI see by your Treehouse profile, you have yet to earn any Python points through coursework. This will make writing Python code difficult unless you have learned basic Python through another source. Perhaps, I can get you started.
When you see a function, such as, f(w)
, this can be defined in Python as:
def f(w):
return 2 * w**3 - 5
Now that function can be called with the argument 4
. Using the interactive python shell:
$ python
Python 3.8.5 (default, Jul 28 2020, 12:59:40)
[GCC 9.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> def f(w):
... return 2 * w**3 - 5
...
>>> f(4)
123
The math functions can be accessed by importing math
, thedatetime
object can be accessed by importing datetime
from math import cos, tan
form datetime import datatime
The other Python commands can be run at the interactive shell prompt (">>>") to see their effect. I can also help with the derivatives, but that a bit beyond this scope.
You may also need
- Setting up a local Python environment (Windows)
- Setting up a local Python environment (Mac)
- Practice Basic Math Calculations in Python
- Python Comprehensions
Post back if you wish more help. Good luck!!

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Pointshello Mr.Chris Freeman i have a few answers, can you tell me if i am close and where i am wrong and what should i do next? i have edited the question section for everyone to see.
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsWhat have you tried? Do you have a more specific question? The forum isn't intended to do one's homework for them.