Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial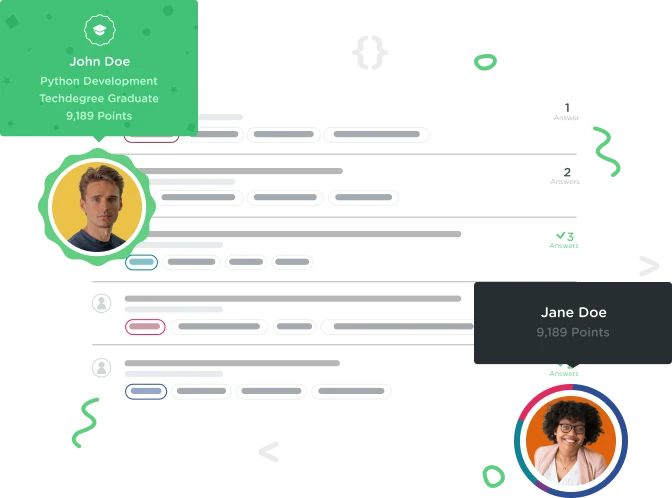

Jay Chin
1,670 PointsProgram always raises ValueError (Python Basics Challenge)
I am currently trying to refine my Master Ticket challenge program. I want the program to loop me back to the confirmation input if one writes anything other than 'y' or 'n'. When I run this, however, no matter what I put in, I will get the error message and I will be looped back to the name portion rather than the confirmation portion. What am I doing wrong here? Any help would be greatly appreciated.
TICKET_PRICE = 10
SERVICE_CHARGE = 2
tickets_remaining = 100
def calculate_price(number_of_tickets):
return number_of_tickets * TICKET_PRICE + SERVICE_CHARGE
while tickets_remaining >=1:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name? ")
num_tickets = input("How many tickets would you like, {}? ".format(name))
try:
num_tickets = int(num_tickets)
if num_tickets > tickets_remaining:
raise ValueError("there are only {} tickets remaining.".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue, {}. Please try again.".format(err))
else:
amount_due = calculate_price(num_tickets)
print("The total due is ${}.".format(amount_due))
try:
should_proceed = input("Do you want to proceed? y/n ")
if should_proceed != "y" or "n":
raise ValueError("Please write only 'y' or 'n'.")
except ValueError as err:
print("{}".format(err))
else:
if should_proceed == "y":
print("Sold!")
tickets_remaining -= num_tickets
else:
print("Thank you anyways, {}!".format(name))
print("Sorry, the tickets are all sold out!")
2 Answers

Jay Chin
1,670 PointsI'm going to answer my own question. It turns out that you can't use "or" with these strings as such. Rather, you have to say that [(should_proceed != "y") and (should_proceed != "n")]
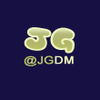
Jonathan Grieve
Treehouse Moderator 91,253 PointsIt might he just an issue of indentation. In your code, indent back your code 4 spaces from the else
keyword inside your while loop. So it looks like this.
TICKET_PRICE = 10
SERVICE_CHARGE = 2
tickets_remaining = 100
def calculate_price(number_of_tickets):
return number_of_tickets * TICKET_PRICE + SERVICE_CHARGE
while tickets_remaining >=1:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name? ")
num_tickets = input("How many tickets would you like, {}? ".format(name))
try:
num_tickets = int(num_tickets)
if num_tickets > tickets_remaining:
raise ValueError("there are only {} tickets remaining.".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue, {}. Please try again.".format(err))
else:
amount_due = calculate_price(num_tickets)
print("The total due is ${}.".format(amount_due))
try:
should_proceed = input("Do you want to proceed? y/n ")
if should_proceed != "y" or "n":
raise ValueError("Please write only 'y' or 'n'.")
except ValueError as err:
print("{}".format(err))
else:
if should_proceed == "y":
print("Sold!")
tickets_remaining -= num_tickets
else:
print("Thank you anyways, {}!".format(name))
print("Sorry, the tickets are all sold out!")
Indentation can cause your program to think it should be running in a different way to how you think it should. Have a play around until you get the behaviour you need. :-)

Jay Chin
1,670 PointsThank you, Jonathan! I ran the code you've provided but it gave me a syntax error on line 26.