Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial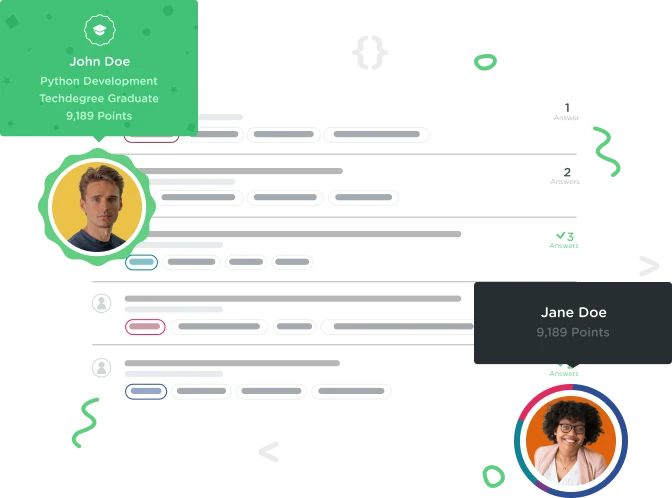
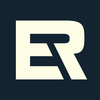
Eric Ridolfi
19,417 PointsPublic X and Y
How does public X and Y get into each of the methods?
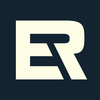
Eric Ridolfi
19,417 PointsI guess I'm just asking a general question dealing with C# objects. I guess I don't understand how in this example the X and Y fields are used in the methods.
using System;
namespace TreehouseDefense
{
class Point
{
public readonly int X;
public readonly int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
public int DistanceTo(int x, int y)
{
/*
int xDiff = X - y;
int yDiff = Y - y;
int xDiffSquared = xDiff * xDiff;
int yDiffSquared = yDiff * yDiff;
*/
return (int)Math.Sqrt(Math.Pow(X-x, 2) + (Y-y, 2));
}
public int DistanceTo(Point point)
{
return DistanceTo(point.X, point.Y);
}
}
}
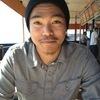
Kevin Agpaoa
10,503 PointsIs your questions referring to the access modifier readonly? I'd like to help, but I need some specifics on your reference to X and Y.
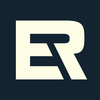
Eric Ridolfi
19,417 PointsMaybe I'm asking the wrong question. In the Point class, the values for X and Y are set from another class, right? Once those values are set it moves on to the Point method. What I don't understand is how the parameters get their values when X is different than x.
1 Answer
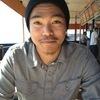
Kevin Agpaoa
10,503 PointsI hope this makes things easier to understand for you. Here it goes: Within class Point it contains two instance variables (X and Y), 1 constructor ( public Point), and two methods that look the same, but taking different parameters.
Using class Point alone, you can initialize a variable to the class Point, for example "Point somePoint". However, the system reserves space for class Point without assigning any values to it. You can pass in values to its constructor.
public Point is like a method, but it's special. It's called a constructor. So when you want assign values to somePoint, you can do it like this
somePoint = new Point(1,2)
The parameters are separate from the variables that Point contains. If you look at the constructor, imagine it's doing this:
What is being passed to the constructor? 1,2 Parameter values: x=1, y=2 What is the constructor assigning those values to? X=x, Y=y
I hope this answers your question.
Edit: I forgot to add. Now that the values X and Y are set, any method within the class can utilize those variables. Also, those values can be accessed outside of the class by somePoint.X or somePoint.Y because of its public modifier.
tobiaskrause
9,160 Pointstobiaskrause
9,160 PointsWhich video/challange??