Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial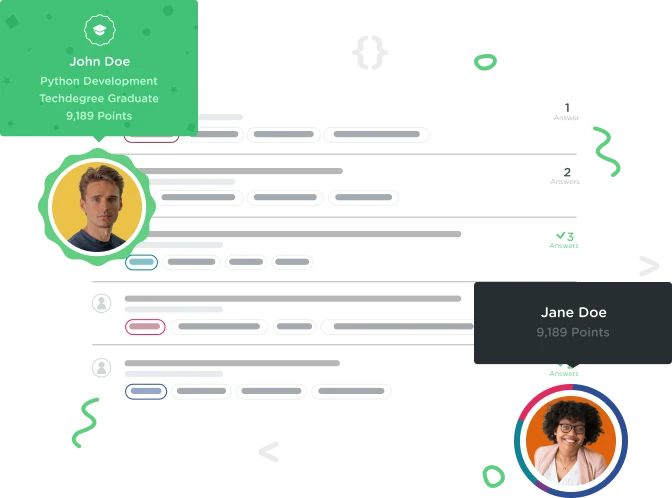
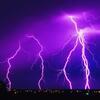
Janelle Mackenzie
7,510 PointsPurpose of the 'val' and 'err' functions?
What is the purpose of the 'val' and 'err' functions? I don't understand how/why those were created..
2 Answers
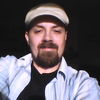
Robert Manolis
Treehouse Guest TeacherHi Janelle, those are actually anonymous functions. The err and val are just parameters that are being passed to anonymous functions. I'll try to talk you through what's happening here.
So when Guil calls breakfastPromise, a "promise" is returned. If you chain a .then or .catch method to the promise, then the value of the returned promise gets passed to those .then or .catch methods. The .then and .catch methods accept an anonymous callback function as an argument. Those callbacks let you perform some operations on whatever value is passed to them from the promise. But you need a way to reference that value being passed, which is accomplished with whatever parameter you pass to the anonymous callback function that was passed to the .then or .catch methods.
I know that probably sounds really confusing. Think about it like this, you can change the names of val and err to anything you want in those callback functions, and the actual value they represent will not be affected, and will still get logged to the console. Give it a try.
Hope that helps shed some light on this.
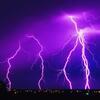
Janelle Mackenzie
7,510 PointsRobert Manolis I understand this much better now, I've been trying to wrap my head around this for a few days. Appreciate your help! :)

Kylie Soderblom
3,890 PointsStill confused. An anonymous function has no name between the function keyword and parentheses ().so it is generally assigned to a variable so you can call it later. var variableName = function () => {console.log (val)} Yet, in this case, there is no word function, no "( )" , no "{ }" and the word 'val' just pops up. val => console.log (val) Could I write function => console.log(val)? still missing the "( )" and the "{ }".
Janelle Mackenzie
7,510 PointsJanelle Mackenzie
7,510 PointsRobert Manolis You mentioned that the var and err were created to allow you to perform operations, and that they are used as a way of reference. But the thing that confuses me is that they were only mentioned once. They were declared at the end of the script and they weren't even a function that served a purpose.. I could understand is var and err were the names of functions that were created and then in the .then and .catch they were being called for performance, but it wasn't even that. They have no purpose its like there was a missing piece of script in this video?
Robert Manolis
Treehouse Guest TeacherRobert Manolis
Treehouse Guest TeacherJanelle Mackenzie - What was passed to the .then and .catch were functions. The following code shows two anonymous functions:
Those functions will log out whatever
err
andval
are. In the case of the video,val
would be whatever is returned from the breakfastPromise Promise. Anderr
would be what ever error that happened to occur while trying to resolve the promise. The log statements are being returned from those anonymous functions to demonstrate how you could do something with theval
returned from the promise and/or theerr
, if one were to occur. Witherr
, you might only want to log it out to the console. But withval
, you could iterate over it, manipulate it, add it to a DOM element and append it to the DOM, etc.The first example above could be changed to something like this:
Hope that clears things up a little more.
karan Badhwar
Web Development Techdegree Graduate 18,135 Pointskaran Badhwar
Web Development Techdegree Graduate 18,135 PointsHi Robert Manolis , what I have understood is that in .then() and .catch() functions we just pass the value of the resolve() or reject() methods, but what happened here when Guil passed Error('Message') Object with the message, to the .catch(), coz it askes to console.log the err message but instead we have passed the error object, so what is happening over there