Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial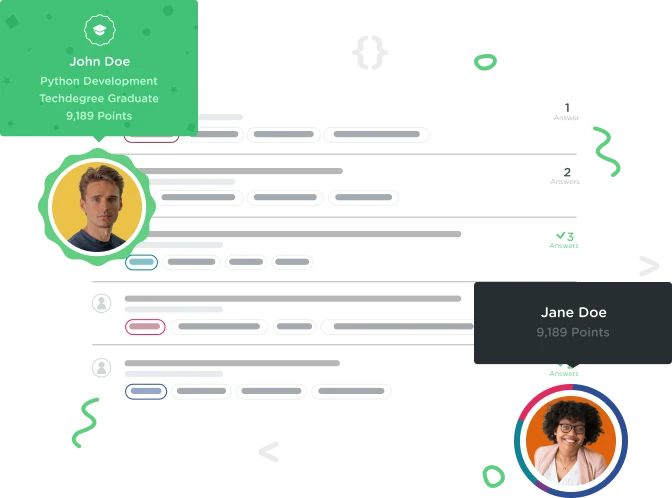
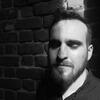
matthew mahoney
Python Development Techdegree Student 2,536 PointsPython Basics: Raising Value Error
Question: I've made a function that creates brand new product names using "artificial intelligence".
I have a problem though, people keep on adding product ideas that are too short. It makes the suggestions look bad.
Can you please raise a ValueError if the product_idea is less than 3 characters long? product_idea is a string. Thanks in advance!
I'm not sure how to check how many characters are in a string... Help?
def suggest(product_idea): return product_idea + "inator"
3 Answers
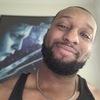
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi matthew,
You can check the length of a string by passing the string in question as an argument to the len()
function.
my_name = βBrandonβ
print(len(my_name))
# output: 7
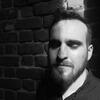
matthew mahoney
Python Development Techdegree Student 2,536 PointsI've made a function that creates brand new product names using "artificial intelligence".
I have a problem though, people keep on adding product ideas that are too short. It makes the suggestions look bad.
Can you please raise a ValueError if the product_idea is less than 3 characters long? product_idea is a string. Thanks in advance!
My Answer: def suggest(product_idea): return product_idea + "inator" if len(product_idea) <= 3: raise ValueError
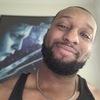
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsOK matthew,
I see the problem. You wrote the logic for your code in the wrong order is all.
Before I get to that I want to inform you that anything after the python interpreter encounters your return statement is unreachable code.
As soon as it evaluated the return statement, the python interpreter exits the function.
So imagine you have a function like this:
def some_func():
return 2
name = βBrandonβ
return name
# the name variable is never created, and 2 is returned from the function
So with the challenge, you need to make sure that you raise the value error before you try to return anything.
Also the product idea has to be less than 3 characters long in order for the value error to be raised. So you donβt want to use the less than or equal to comparison operator in this situation. Unless your test is the following:
if len(product_idea) <= 2: ...
def suggest(product_idea):
if len(product_idea) < 3:
raise ValueError()
return product_idea + "inatorβ
Hope that helps.
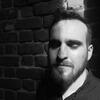
matthew mahoney
Python Development Techdegree Student 2,536 PointsWow, thank you so much for the time you invested in helping me. I really appreciate your help. Got it!! Thanks.
matthew mahoney
Python Development Techdegree Student 2,536 Pointsmatthew mahoney
Python Development Techdegree Student 2,536 PointsThanks, soo much!
matthew mahoney
Python Development Techdegree Student 2,536 Pointsmatthew mahoney
Python Development Techdegree Student 2,536 PointsI'm still not passing the quiz... what am I doing wrong?
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsPost your code here and Iβll evaluate it for you. Tell you where the bug may lie.