Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial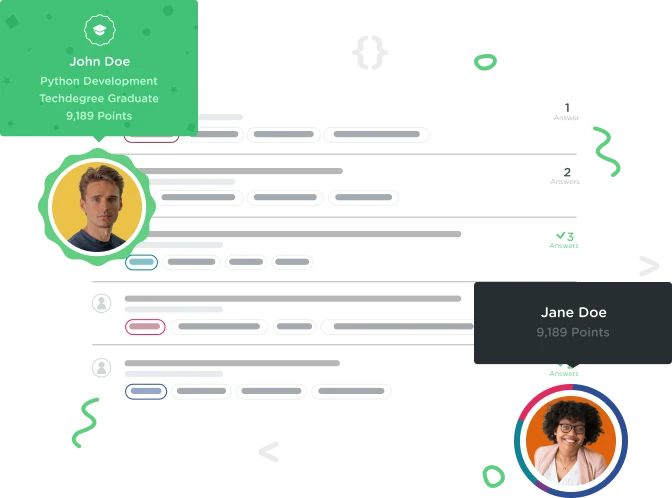
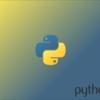
Kevin Faust
15,353 Pointspython csv workshop help
hi,
import csv
with open('museum.csv', newline='') as csvfile:
artreader = csv.reader(csvfile, delimiter='|')
rows = list(artreader)
for row in rows[1:3]:
print(', '.join(row))
can someone explain what newline='' and delimiter='|' do?
what does artreader contain after we do csv.reader(...)?
since i dont have the museum.csv file, it is hard for me to understand any of this
3 Answers
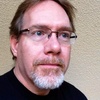
Chris Freeman
Treehouse Moderator 68,426 PointsFrom the CSV docs:
> can someone explain what newline='' and delimiter='|' do?
Files should be opened with newline=''
. If newline=''
is not specified, newlines embedded inside quoted fields will not be interpreted correctly, and on platforms that use \r\n
linendings on write an extra \r
will be added. It should always be safe to specify newline=''
, since the csv module does its own (universal) newline handling. (src)
The delimiter is the character separating the fields in the line of text. delimter='|'
means "`use the pipe character (|) as the marker between the fields. Defaults to comma. (src)
> what does artreader contain after we do csv.reader(...)?
csv..reader()
Return a reader object which will iterate over lines in the given csvfile (src). When wrapped by the list()
function, it will create a list of all the rows in the file where each row is a list of all the elements.

David Wright
4,437 PointsGreat description, Chris! Thanks!

Tonye Jack
Full Stack JavaScript Techdegree Student 12,469 PointsAnother way to write csv from files like JSON file
with open('testfile.csv', 'w') as csvfile:
fieldnames = ['Id', 'Firstname', 'Lastname', 'Course']
testwriter = csv.DictWriter(csvfile, fieldnames=fieldnames, delimeter='|')
testwriter.writeheader()
data = {
'firstnames': ['Tom', 'James', 'Johnes', 'Jeremy'],
'lastnames': ['Jay', 'Timmy', 'Tommy', 'Jill'],
'courses': ['Python', 'Java', 'Web', 'Graphics']
}
for i, v in enumerate(data['firstnames'], start=1):
testwriter.writerow({
'Id': i,
'Firstname': v,
'Lastname': data['lastnames'][i-1],
'Course': data['courses'][i-1]
})
Kevin Faust
15,353 PointsKevin Faust
15,353 Pointsi still dont get newline='' and delimeter. i found a sample csv file online and the results with/without newline='' are the same. same with delimeter
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsThe difference is the End-of-Lines between the
\r\n
(PC) and the\n
(Mac/Linux). If you are on Mac or Linux, usingnewline
or not would be the same.Depending on the file you chose, if the delimiter in the file is a comma, then specifying the delimiter argument shouldn't matter. If you are specifying a different delimiter than that used in the file, the results should differ.