Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial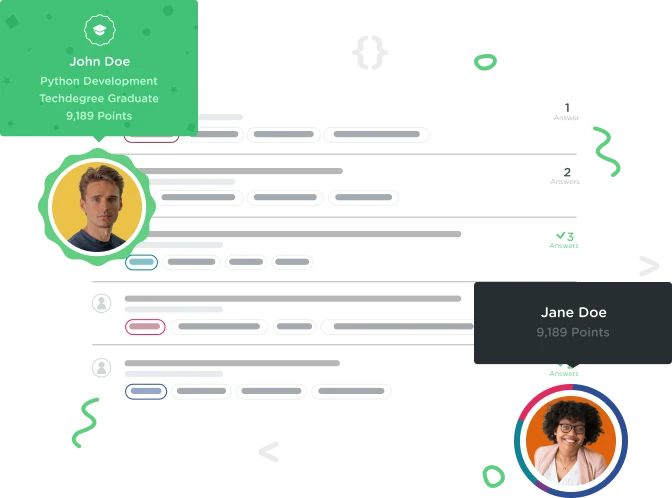

Enes artun
Courses Plus Student 2,364 PointsPython function exercises
Hi. I have been working one some fucntion exercises and I need help on some of them.
Write a Python function that checks whether a passed string is a palindrome or not.
Note: A palindrome is a word, phrase, or sequence that reads the same backward as forward, e.g., madam or nurses run.
def isPalindrome(string):
left_pos = 0
right_pos = len(string) - 1
while right_pos >= left_pos:
if not string[left_pos] == string[right_pos]:
return False
left_pos += 1
right_pos -= 1
return True
print(isPalindrome('aza'))
I don't understand this one. Would appericiate an explanation.
Write a Python function to check whether a number falls within a given range.
def check_number(x,y,num):
for num in range(x,y):
if num>x and num<y:
print(f'Number {num} in range')
check_number(2,6,4)
This is my code. When I run it, I get number 2 in range, number 3 in range etc instead of one number in range. How can I fix it?
def test_range(n):
if n in range(3,9):
print( " %s is in the range"%str(n))
else :
print("The number is outside the given range.")
test_range(5)
This is the answer to that question.
Thnak you!
1 Answer
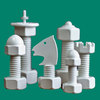
Steven Parker
230,995 PointsThe first code uses a loop to compare the front part of the string to the end. If the first character matches the last, it moves on to compare the 2nd to the 2nd-to-last, and so on. If any don't match, it returns False. The loop ends when the pointers to both ends meet in the middle, and then the function returns True.
In the second sample code, the range is being set to cover 2 through 6, so it is correct for both 2 and 3 to be reported in range. What made you think it wasn't working and needed to be fixed?
In the third code, the custom test_range function that has a fixed range of 3 through 9 also seems to work fine. If I run it as-is, I get 5 is in the range
. But if I call test_range(2)
instead, I get The number is outside the given range.
.
And I'm not sure what your last sentence "This is the answer to that question." is referring to.
Enes artun
Courses Plus Student 2,364 PointsEnes artun
Courses Plus Student 2,364 PointsThank you for the explanation. Question says a number. When I run my code, I get all the numbers between that range.
def test_range is the answer of the second question. Write a Python function to check whether a number falls within a given range.
Steven Parker
230,995 PointsSteven Parker
230,995 PointsOK, I get it now. The second code has a loop that overwrites "num" with the values from the range. If you remove this line:
for num in range(x,y):
Then instead of looping, it will test the "num" given as an argument.
Also if you want the range to be inclusive, change the comparisons to allow equality:
if num >= x and num <= y: