Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial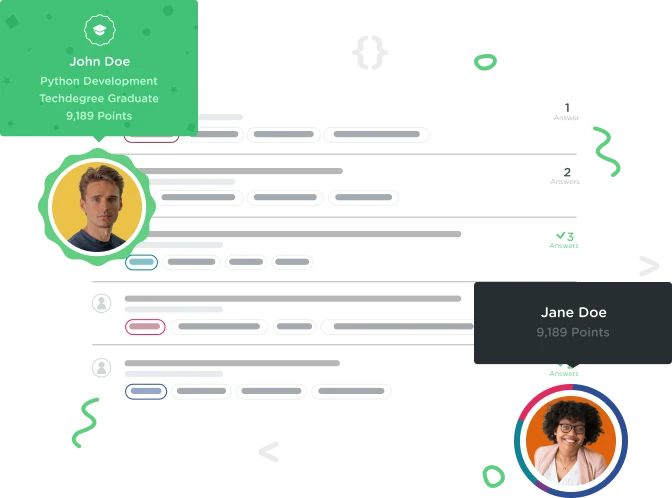
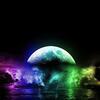
rubiks69
1,647 PointsPython project: 4x4 matching game. No cards matching and doesn't tell you what the cards are. Pls help.
My problem is that there are never any matches to any of the guesses I put in and when I get it wrong it doesn't tell you what the cards were. if it makes any difference am using the python app and not the treehouse workspace.
Here is the code for the game.py file:
from cards import Card
import random
class Game:
def __init__ (self):
self.size = 4
self.card_options = ["dev", "aim", "fin", "vex", "zip", "orb", "ice", "eel"]
self.columns = ["a", "b", "c", "d"]
self.cards = []
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f"{column}{num}")
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f"{column}{num}":
if card.matched:
row.append(str(card))
else:
row.append("===")
return row
def create_grid(self):
header = "=|==" + "==|==".join(self.columns) + "==|"
print(header)
for row in range(1, (self.size + 1)):
print_row = f"{row}|="
get_row = self.create_row(row)
print_row += "=|=".join(get_row) + "=|"
print(print_row)
def check_match(self, location1, location2):
cards = []
for card in self.cards:
if card.location == location1 or card.location == location2:
cards.append(card)
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f"{card.location}: {card}")
return False
def check_win (self):
for card in self.cards:
if card.matched == False:
return False
else:
return True
def check_location(self, time):
while True:
guess = input(f"What's the location of your {time} card?")
if guess.lower() in self.locations:
return guess.upper()
else:
print("That's not a valid location. It should start with a, b, c or d and end with a number from one two four")
def start_game(self):
game_running = True
print("Memory Game")
self.set_cards()
while game_running:
self.create_grid()
guess1 = self.check_location("1st")
guess2 = self.check_location("2nd")
if self.check_match(guess1, guess2):
if self.check_win():
print("Congrats!!! You won the game.")
self.create_grid
game_running = False
else:
input("Those cards don't match =(. Press enter to continue")
print("Game Over")
if __name__ == "__main__" :
game = Game()
game.start_game()
And here is the code for the cards.py file:
class Card:
def __init__ (self, word, location):
self.card = word
self.location = location
self.matched = False
def __eq__ (self, other):
return self.card == other.card
def __str__ (self):
return self.card
1 Answer
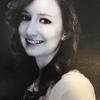
Megan Amendola
Treehouse TeacherThere are a couple of things going:
- In
check_location
you need to returnguess.lower()
and notguess.upper()
. You have saved your locations as lowercase values and this is why you're not getting any matched cards because no locations are matching. Thecheck_location
is returning A1 but your locations are lowercase like a1 so nothing matches. (Fix this first and run to see the error you get next) - Inside of check match, your indentation is off. Here's what it should look like:
def check_match(self, location1, location2):
cards = []
for card in self.cards:
if card.location == location1 or card.location == location2:
cards.append(card)
#This is checking the cards at the two locations to see if they match
# this needs to be outside of the loop or it will through an error
if cards[0] == cards[1]:
cards[0].matched = True
cards[1].matched = True
return True
else:
for card in cards:
print(f"{card.location}: {card}")
# this also needs to be outside the for loop
# return automatically stops what is happening so if it's inside the loop
# it will only show the first value and then return False
return False
rubiks69
1,647 Pointsrubiks69
1,647 PointsThank you! it all works now