Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial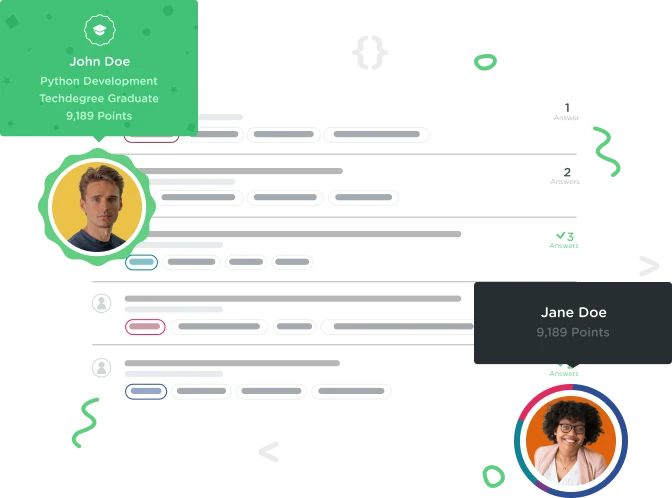
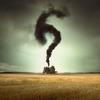
Jordan Barr
21,335 PointsRails bloodhound + typeahead autocomplete
Hi all,
Im looking for anyone who can help give me some guidance on implementing a "drop down" suggestion box for a project I'm working on. I am using Rails 5.1 with Searchkick (Elasticsearch) and am trying to implement the twitter bloodhound/typeahead to give a drop down menu of searchable items. For reference and if it helps to visualise things, here is a link to my project website: https://immunophenotyping.online
Anyway ill add code snippets / comments below and if anyone is able to help or provide ideas I would greatly appreciate it :)
routes.rb - This is my default route when you first land on the page and I'm assuming that the autocomplete part of the route needs to be appended here in a collection. I assume this has to link back to the controller action 'autocomplete' defined below, but unsure if I have done this correctly in the routes file.
root 'welcome#index' do
collection do
get :autocomplete
end
end
welcome_controller.rb - autocomplete method inside my welcome controller - Obtained from the Searchkick docs on GitHub: https://github.com/ankane/searchkick#instant-search--autocomplete
def autocomplete
render json: Cell.search(params[:q], {
fields: ["name^5"],
minLength: 2,
autocomplete: true,
limit: 5,
load: false,
misspellings: false
}).map(&:name)
end
input field/search box
<%= form_tag(@Search, :action => 'index', :method => "get", id: "search-form typeahead") do %>
<%= text_field_tag(:q, nil, placeholder: "Search...", class: "form-control rounded-left typeahead", autocomplete: "off", id: "typeahead") %>
<% end %>
Firstly I'm unsure if I am even putting the javascript in the correct place, or if I should be placing it all in application.js file.
index.html javascript -- Very confused about setting the source as I think this links back to the controller method? Sadly the docs were a little confusing: https://github.com/twitter/typeahead.js/blob/master/doc/bloodhound.md
<script>
var autocomplete = new Bloodhound({
datumTokenizer: Bloodhound.tokenizers.whitespace,
queryTokenizer: Bloodhound.tokenizers.whitespace,
remote: {
url: '/welcome/autocomplete?q=%QUERY',
wildcard: '%QUERY'
}
});
$('#q').typeahead(null, {
source: autocomplete
});
</script>
application.js -- As stated above, confused about the 'source' as I think this should be pointed at the controller method, but do I have my method in the correct controller?
var autocomplete = new Bloodhound({
datumTokenizer: Bloodhound.tokenizers.whitespace,
queryTokenizer: Bloodhound.tokenizers.whitespace,
remote: {
url: '/welcome/autocomplete?q=%QUERY',
wildcard: '%QUERY'
}
});
$('#typeahead').typeahead({
minLength: 2,
highlight: true
},
{
name: 'autocomplete',
source: autocomplete
});
Anyway as you can see, very confused about how to go about this and all other online resources (that I have found) seem to be outdated. Thank you for taking the time to read / look over this, I really do appreciate any help :)
EDIT: formatting & added links to docs