Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial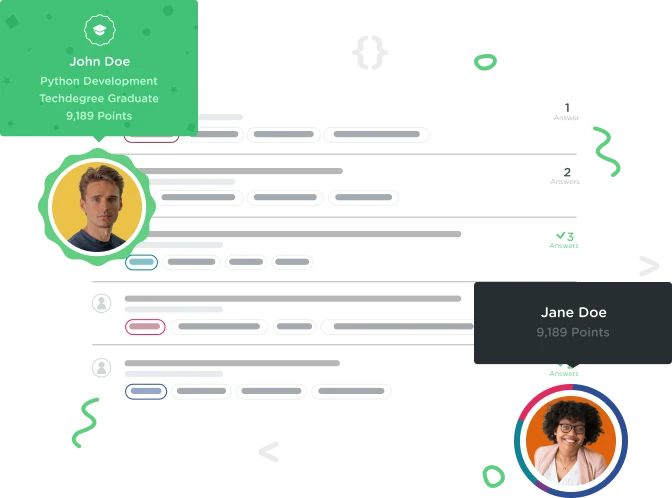

mattmilanowski
15,323 PointsRepeating this course outside of workspaces using Visual Studio
It seems like there are a lot of questions about doing this course outside of workspaces. I see how workspaces can normalize everyone's experience and that's great for teaching the material. I feel like a lot of students though realize that learning this database course isn't very valuable to them unless they understand how to set up a database of some sort outside of the workspaces. I'm in that same mindset.
I've been trying to use Visual Studio with the Python Extension and Sqlite to get me out into the 'wild'. Pip is already installed in Visual Studio and I did get peewee installed and updated. I believe I'm on the right track. I'm running into a few problems still just trying to get similar code to the teacher's to run without errors though.
Here's what I've got:
class students(object):
from peewee import SqliteDatabase, Model
from peewee import CharField, DateTimeField, BooleanField, TextField, ForeignKeyField, IntegerField
from peewee import IntegrityError
db = SqliteDatabase('Students.db')
class Student(Model):
username = CharField(max_length=255, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
if __name__ == '__main__':
db.connect()
db.create_tables([Student], safe=True)
My first issue where the course was not matching my experience in Visual Studio was the statement from peewee import *
This didn't work. The error was: 'import * only allowed at module level'. I read in another thread that I could import the things I need specifically, so I did. You can see all my 'from peewee import' statements above.
I'm still running into issues starting the program in Visual Studio. It errors out at the username = CharField(max_length=255, unique=True)
line. The error is "The name "CharField" is not defined'
I'm about out of ideas. I thought I defined it in the 'from peewee import CharField' statement. Any suggestions? I'd also be up for arguments against using Visual Studio for this, and better real world alternatives for using Windows, Python and Databases, happily together. I suppose I'll head over to Django courses now to see what that's all about.
6 Answers

Jo S
3,073 PointsThere are several issues in your code:
1) your name == 'main' if statement is incorrectly indented.. 2) your db = SqliteDatabase call is in the wrong place in your module 3) Your peewee import statement should be in the same file as your SqliteDatabase code
There may also be an issue with the following, but I cannot test that as I dont have Visual Studio 4) incorrect installs of peewee or python tools for visual studio
i suggest fixing issues 1)-3) before considering whether it's another problem

mattmilanowski
15,323 PointsOk I tried a couple things and got it to function correctly. I moved everything out of the separate students.py file I created to store students class. I moved all code back to the file I initially created in Visual Studio called PythonDatabaseCourse.py. In that py file I now have the Student Class. Everything works like normal. I added all the rest of the code from the end of this part of the course and everything works find.
from peewee import *
#from peewee import SqliteDatabase, Model
#from peewee import CharField, DateTimeField, BooleanField, TextField, ForeignKeyField, IntegerField
#from peewee import IntegrityError
db = SqliteDatabase('Students.db')
class Student(Model):
username = CharField(max_length=255, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
students = [
{'username': 'kennethlove',
'points': 154888},
{'username': 'chalkers',
'points': 11912},
{'username': 'joykesten2',
'points': 7363},
{'username': 'craigdennis',
'points': 4079},
{'username': 'davemcfarland',
'points': 14747}
]
def add_students():
for student in students:
try:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
student_record = Student.get(username = student['username'])
student_record.points = student['points']
student_record.save()
def top_student():
student = Student.select().order_by(Student.points.desc()).get()
return student
if __name__ == '__main__':
db.connect()
db.create_tables([Student], safe=True)
add_students()
print("Our top student right now is : {0.username}.".format(top_student()))
My big hang up here the whole time is my lack of understanding about how to break out classes into different .py files in Visual Studio and then call them from the main py file. I'll have to do more research on that topic but hopefully this helps future students interested in giving Visual Studio a try. Thanks for playing along, Jo S!

Jo S
3,073 PointsI think you placed the import statements in the wrong spot. import statements should be placed at the very beginning of your file (or module), ie at 'module level'.
Your import statements are inside the class Student block and indented. Take them out and place them on the first line of your file.
That should allow you to use "from peewee import *" as well.
I don't have Visual Studio to test this, but pls let me know if it worked.

mattmilanowski
15,323 PointsOk, I removed all my specific statements from the students class. The main py file I start with I called PythonDatabaseCourse.py. That's where I'd call the students class and start the program. Here's what is in that py file now:
from peewee import *
import students
When I run the program now, it bounces me to the students class and on this line:
db = SqliteDatabase('Students.db')
It now gives me an error saying 'name 'SqliteDatabase' is not defined' Seems like it doesn't know about the 'from peewee import *' line in my other file.

Jo S
3,073 PointsWhat exactly do you mean by it bounces me to the students class? And why are you importing students? Where is the db = SqliteDatabase line located in your code?
It's probably easiest if you post the full code and the full error message to clarify

mattmilanowski
15,323 PointsIt bounces just means it flipped over to the students.py file tab in Visual studio.
Full Code: There is a file called PythonDatabaseCourse.py. It contains this code:
from peewee import *
import students
Then there is another file called students.py. It contains this code:
class students(object):
#from peewee import SqliteDatabase, Model
#from peewee import CharField, DateTimeField, BooleanField, TextField, ForeignKeyField, IntegerField
#from peewee import IntegrityError
db = SqliteDatabase('Students.db')
class Student(Model):
username = CharField(max_length=255, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
if __name__ == '__main__':
db.connect()
db.create_tables([Student], safe=True)
That's it. I put import students
in the PythonDatabaseCourse.py file because that's the file that seems to run when I push the Start button in Visual Studio. Nothing really happens if I don't put anything in the main file so I just thought calling the class I wanted to run was the correct thing to do.
My error after pressing the play/start button in VS is 'NameError was unhandled by user code' 'name "SqliteDatabase" is not defined."
So maybe my problem like you said, is that I didn't load or configure Sqlite in any way in Visual Studio. I'll look into that.
In other news I downloaded PyCharm, watched the Treehouse PyCharm workshop, and am going through this database course with no problem, so far in PyCharm.

Jo S
3,073 Pointsalso why do you have this line?
class students(object):

Jo S
3,073 PointsI am glad this worked. I deliberately didn't give you full explanations about what the specific errors are and what the script should be explicitly so that you can research it by yourself.
I suggest you look up these things:
1) if name == 'main': what does this mean/do?
2) what does indentation mean/do in python?
You can look it up on Google or rewatch the basic python modules from Kenneth, I'm pretty sure he goes through the explanations - but I can't remember exactly in which videos.
mattmilanowski If you don't mind, could you pls select my previous comment as the Best Answer ? Thanks!