Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial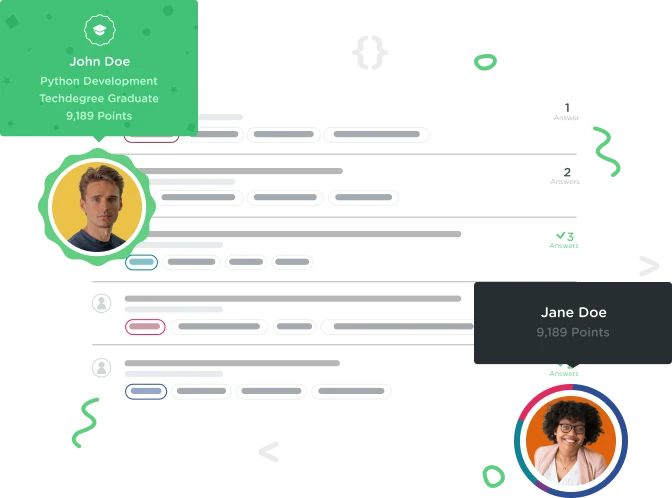

Derek Etnyre
20,822 PointsReplace the accessor methods - not quite right.
Programming looks like this:
namespace Treehouse.CodeChallenges
{
public class Frog
{
private NumFilesEaten _numFliesEaten;
Public NumFliesEaten FliesEaten
{
get
{
return _numFliesEaten;
}
set
{
_numFliesEaten = value;
}
}
}
}
suspect this line is the problem:
Public NumFliesEaten FliesEaten
but not sure what I should use in place of FliesEaten?
Any advice to get me going in the right direction?
namespace Treehouse.CodeChallenges
{
public class Frog
{
private NumFilesEaten _numFliesEaten;
Public NumFliesEaten FliesEaten
{
get
{
return _numFliesEaten;
}
set
{
_numFliesEaten = value;
}
}
}
}
7 Answers
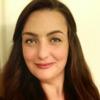
Jennifer Nordell
Treehouse TeacherOh wow! You're so close, Derek Etnyre ! First, and foremost, you've misspelled the keyword "public". Secondly, you don't need anything in place of the FliesEaten. Also, we're going to be returning an int, which you never state. Getters and setters have an implicit parameter called "value". So my solution looks like this:
namespace Treehouse.CodeChallenges
{
public class Frog
{
private int _numFliesEaten;
public int NumFliesEaten
{
get
{
return _numFliesEaten;
}
set
{
_numFliesEaten = value;
}
}
}
}
Documentation about the implicit parameter value can be found on the MSDN here:
https://docs.microsoft.com/en-us/dotnet/csharp/language-reference/keywords/value
Hope this helps!
edited to update the documentation to the current URL

Derek Etnyre
20,822 PointsThank you for the help - your explanation was really good.
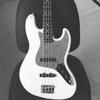
Lee Puckett
12,539 PointsThanks for the explanation. I think the video and challenge are confusing because in the video there is no "int" used in the original code, but there is in the challenge. Also in the challenge there are underscores in the original code whereas there are no underscores in the video example, so that threw me off, especially since I'm not sure what their purpose is and when to use or not use them.
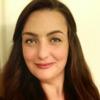
Jennifer Nordell
Treehouse TeacherThe use of the underscore is to denote a private field or member. Some consider it to be a good naming convention and others don't. It will largely depend on what your team decides if you work with one. In my opinion, I sort of like it. It makes it immediately obvious that the property we're talking about is private.
As for the video example, I'm wondering if we're looking at the same video. Because there are underscores throughout the video preceding the challenge that's linked to this question. Take a look at 1:23 of this video.
The int
is simply the data type of the thing being returned. In the video, what we're returning is an instance of MapLocation
which is one of our custom classes. But this example isn't nearly as complex. If we had needed to return a string, then it would've been public string
. The thing after public is just denoting the data type we're returning .
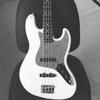
Lee Puckett
12,539 PointsJennifer Nordell
Thanks. I now see where he got _location from now. Thanks! I still thing using a challenge with int is confusing because it lights up a different color, which would lead me to think that it is in a different category than MapLocation, but you are saying int and MapLocation serve the same function (?).
A separate question:
At :42 in the video, he says the type should match the type of the field. He copies MapLocation to line 17 and adds the name Location. In your example above you did not match. You wrote public int NumFliesEaten even though the original was private int _numFliesEaten. I understand this is because of the underscore rule making something private but if that's the case, why wasn't there an underscore before MapLocation in the video? Instead it reads: private MapLocation, _location.....with no underscore.
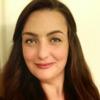
Jennifer Nordell
Treehouse TeacherHi there! I feel obliged to point out here that I think you might be mistaking me for Treehouse staff. I am not. I'm simply a student just like yourself. If you'd like to send feedback to Treehouse about how this challenge could be made better, I strongly encourage you to contact them directly by mailing help@teamtreehouse.com
I think you're confusing the data type of the return with the function name and variable names. The MapLocation
is a data type. We are sending back a private member named _location
with the type of MapLocation
. This is the name of the method. He chose "Location" as the name, but he could've chosen anything really. And it does match this example as the name of the method in this example is NumFliesEaten
.
The reason that int
becomes a different color is that it is a built-in data type. The MapLocation
type was a custom made data type by us.
Yes, he says that the type of the return should match the type of the field. The type of the field in the video is MapLocation
and the type returned is MapLocation
. This is also true of this example. The type of the field is int
and the return type is also int
.
The variable is private which is exactly why we need a public getter and setter. We need code from outside this class to be able to change it, but by encapsulating it this way, it makes it harder to do on accident.
Let's take a look line for line.
public class Frog //declare a public class Frog
{
private int _numFliesEaten; //declare a private integer variable
//Note here that int is the type of the variable
public int NumFliesEaten //declare a function that will get and set the value of the above variable of type int
//Note here that int is the type being returned by the function and the name of the function
{
get
{
return _numFliesEaten; //return an integer to read the value stored in our private variable
}
set
{
_numFliesEaten = value; //allow for the assignment of an integer to our private variable
}
}
}
Hope this clarifies things!
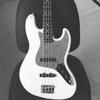
Lee Puckett
12,539 PointsThank you for your help.
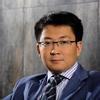
Andrei Li
34,475 PointsMy question is regarding a "value" variable. I can name it as I want. Is it correct? Can it be anything? Like "value1".
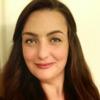
Jennifer Nordell
Treehouse TeacherNo, you may not. The value
, as stated before, is an implicit variable(or keyword) defined by C#. Here is some MSDN documentation
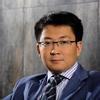
Andrei Li
34,475 PointsThank you, Jennifer!
Lee Puckett
12,539 PointsLee Puckett
12,539 PointsHow is Public misspelled?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi Lee Puckett! I wrote this just over a year ago. I probably should have said "improperly capitalized"- Everything is case-sensitive in C#. He had typed
Public
when he meant to typepublic
.Lee Puckett
12,539 PointsLee Puckett
12,539 PointsThanks for the explanation. I think the video and challenge are confusing because in the video there is no "int" used in the original code, but there is in the challenge. Also in the challenge there are underscores in the original code whereas there are no underscores in the video example, so that threw me off, especially since I'm not sure what their purpose is and when to use or not use them.