Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial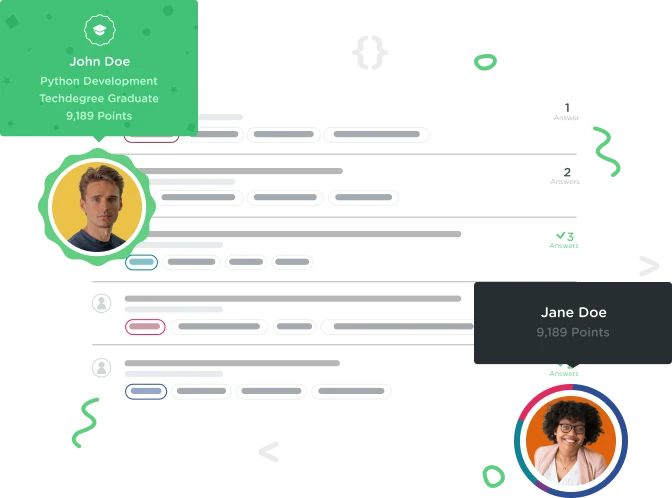

Rulon Taylor
2,762 PointsRepost because code didn't post: TreeStory2. Why does filtering work in the IDE but not the challenge?
My code runs fine in the IDE, the filter catches dork and other filtered words. However, when I paste the same code in the challenge, it gives me an error stating that dork somehow gets through. Can anyone explain please?
Adding code here since it failed to post again:
Main.java
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = null;
try {
story = prompter.promptForStory();
} catch (IOException ioe) {
ioe.printStackTrace();
}
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
Prompter.java
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println("Your TreeStory:");
System.out.printf(tmpl.render(results));
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
String response = "";
do {
System.out.printf("Please enter a %s%n", phrase);
response = mReader.readLine();
if (mCensoredWords.contains(response)) {
System.out.printf("Sorry %s is not allowed.%n", response);
}
} while (mCensoredWords.contains(response));
return response;
}
public String promptForStory() throws IOException {
System.out.println("Enter a fill in the blank story with a double underscore on each side of the blanks to be filled.");
return mReader.readLine();
}
}
[MOD: added ```java markdown formatting -cf]
1 Answer

Rulon Taylor
2,762 PointsThanks for the comment Chris.
I figured the problem out this morning. I was printing a message echoing back the censored word. The testing software was blindly looking at all output for an instance of the word rather than looking only at the final program output. I removed the echo message, and suddenly passed the challenge.
Good reminder that automated code test is not intelligent code test. :)
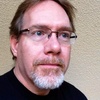
Chris Freeman
Treehouse Moderator 68,441 PointsMoved comment to answer.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsIs it a case-sensitive issue? "dork" vs "Dork"?