Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial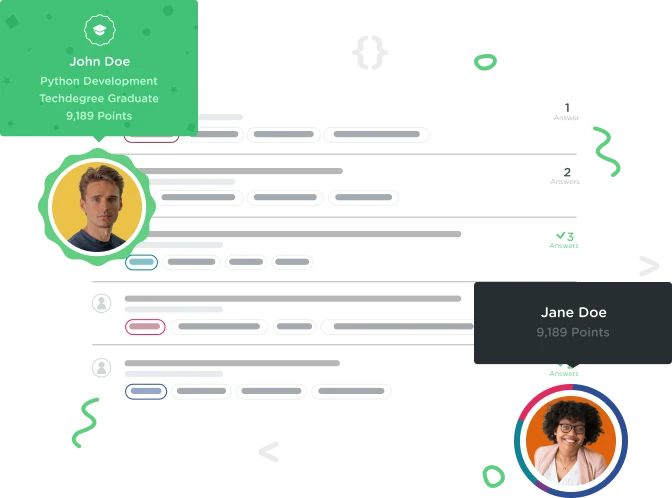

sammihsieh
1,934 Pointsreturn a string that says 'Bao Bao eats bamboo.' where 'Bao Bao' is the name attribute and 'bamboo' is the food attribut
Challenge Task 2 of 3 Create a method called eat. It should only take self as an argument. Inside of the method, set the is_hungry attribute to False, since the Panda will no longer be hungry when it eats. Also, return a string that says 'Bao Bao eats bamboo.' where 'Bao Bao' is the name attribute and 'bamboo' is the food attribute. Please help...
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
name = "Bao Bao"
return (f'{name} eats {food}.')
2 Answers
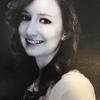
Megan Amendola
Treehouse TeacherYou should be using the name instance attribute so a name can be passed in for the Panda and returned. Right now you are creating a name variable inside of the eat method and setting it equal to Bao Bao, so if the user wants to name their Panda "Charles", they will have the wrong name returned to them.
You also don't need the () in the return statement.
def eat(self):
self.is_hungry = False
return f'{self.name} eats {self.food}.'

Joseph Haverty
8,168 PointsI just ran into the exact same problem. I had to re-attribute name and food their specific values inside the eat function def eat(self): self.is_hungry = False name = 'Bao Bao" food = 'bamboo' return f'{self.name} eats {self.food}.'
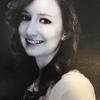
Megan Amendola
Treehouse TeacherWhen a panda instance is created, a name and age have to be passed, for example:
panda = Panda('Charles', 2)
Inside of dunder init, it takes the name that was passed in and sets it as an instance attribute. Inside of eat, you are accessing the name instance attribute and the class food attribute to return the message.
return f'{self.name} eats {self.food}.'
So for this instance, it would return 'Charles eats bamboo' when panda.eat()
is called.
I could create a different instance panda2 = Panda('Nia', 5)
and when I call panda2.eat()
I should then get 'Nia eats bamboo'.