Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial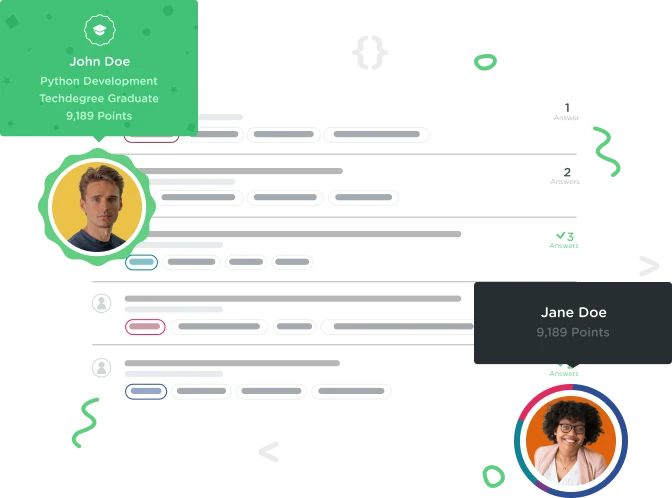
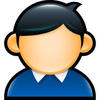
Daniel Hildreth
16,170 PointsShorthand Operators
I am still getting a little confused on the shorthand operators. For instance, in the C# basics course, it had "RunningTotal = RunningTotal + minutes". It was shortened to "RunningTotal += minutes". I need help understanding how this is working. Is it saying RunningTotal + minutes = Running Total, as if the = sign is taken out and put back in at the end? I might be overthinking this, but I need help breaking it down, so I can understand it better.
3 Answers

Christian Andersson
8,712 PointsThe +=
shorthand operator, commonly called the "Addition Assignment Operator", basically means "increment the current value of this variable by...`.
Example:
result += 5
This will increase the result by 5. If the original value was 9, it will now be 14.
Take a look at the official documentation here
There are other common such variables, such as -=
, *=
and so on. Good luck.

Damien Watson
27,419 PointsHi Daniel,
It can be a little confusing at first, but there are a few of these shorthand operators. As Christian has said. You can also use this to concatenate strings (I believe C# is the same).
As you initially said var = var + otherVar
was replaced with var += otherVar
. This is shorthand and it saves you a few characters, also makes your code a bit more compact.
In mathematical operations, you can also use -=
, *=
and /=
.
With +=
, the variable on the left equal to itself +
the variable on the right. The easiest way to explain might be some basic examples:
abc = 10;
def = 5;
abc += def;
// abc = abc + def
// abc = 10 + 5
// abc = 15
abc -= def;
// abc = abc - def
// abc = 15 - 5
// abc = 10
abc *= def;
// abc = abc * def
// abc = 10 * 5
// abc = 50
abc /= def;
// abc = abc / def
// abc = 10 / 5
// abc = 10
I hope this helps, happy to clarify if you need.

Vance Rivera
18,322 PointsThis may be a little confusing at first but it is pretty straightforward. The += just means you are adding variable to itself plus another variable. The shorthand allows you to omit writing out the whole statement.
int counter = 1;
counter = counter + 2;
//evaluates the same as
counter += 2;