Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial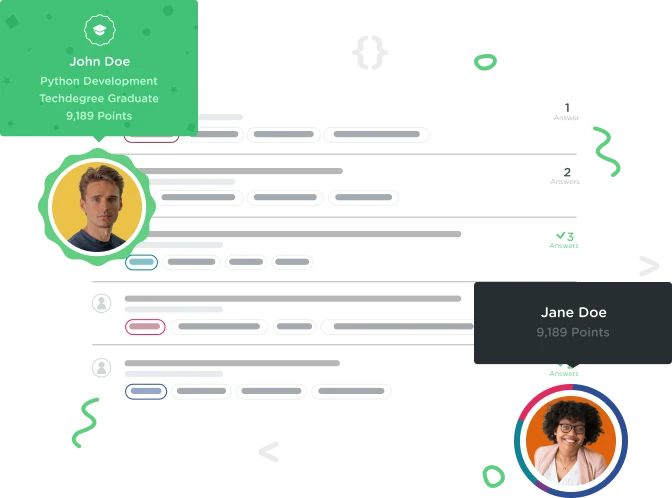

TJ Takei
13,362 PointsShould we exclude the top title out of the prerequisite set?
Is the top item's title 'Django Basics' expected not to be in the output of prereqs()? The following code fails because of that, or else?
courses = {'count': 2,
'title': 'Django Basics',
'prereqs': [{'count': 3,
'title': 'Object-Oriented Python',
'prereqs': [{'count': 1,
'title': 'Python Collections',
'prereqs': [{'count':0,
'title': 'Python Basics',
'prereqs': []}]},
{'count': 0,
'title': 'Python Basics',
'prereqs': []},
{'count': 0,
'title': 'Setting Up a Local Python Environment',
'prereqs': []}]},
{'count': 0,
'title': 'Flask Basics',
'prereqs': []}]}
def prereqs(data, pres=None):
pres = pres or set()
pres.add(data['title'])
return reduce(lambda acc, x: prereqs(x, acc), data['prereqs'], pres)
2 Answers
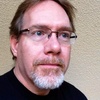
Chris Freeman
Treehouse Moderator 68,426 PointsYes. "Django Basics" is not a prerequisite.
Instead of adding title then reducing, try doing for each prerequisite add then reduce.

TJ Takei
13,362 PointsThank you, Chris! Yes, that's it!
TJ Takei
13,362 PointsTJ Takei
13,362 PointsThanks, Chris. Now I changed as below to drop the top title, alas it fails. Why?
[MOD: added ```python markdown formatting -cf]
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsYou code passes for me... after I remember to import
reduce
from functools import reduce
You code is running a double recursion in that it creates a new a function using lambda then calls
prereqs
using that function. It can be unrolled by using afor-loop
:Here is my alternative solution.
Joshua Yoerger
11,206 PointsJoshua Yoerger
11,206 PointsChris, I am a bit confused by the line
pres = prereqscf(course, pres)
in your alternate solution below - specifically, with how the syntax corresponds to what Python is doing behind the scenes. I have stepped through the code line by line a few times trying to wrap my head around the (double) recursion and I noticed that the variable
pres
always points to theset object
and never to instances of thefunction object
. I also noticed that removing the assignment from that line - i.e.prereqscf(course, pres)
does not seem to change the behavior of the program at all. If you could shed any light on this for me, I would be very grateful! Thanks.
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsJoshua, Yes, the two variations do provide the same results. The difference between assigned the recursive call to
pres
or simply callingprereqs()
is more about clarity of coding.Since
pres
is a set (container), when it is passed as an argument, certain operations will operate on the container in-place and not create a local version of the variable.set.append()
is one of these functions. So when the set is appended, it is affectingpres
everywhere since they are all pointing to the same object.Because the function returns
pres
, the local value within this iteration will already be the same as the returned value making the assignment redundant. For clarity of coding, if a recursive function returns a value, I prefer to see how that value is used explicitly verses having to realize that the recursive call has a side-effect of updatingpres
.This is a good practice to follow since not all objects will behave the same as a
set
in a recursive manner (such as string operations).Adding a
print
to show the "id" ofpres
it will show that the object is the same throughout the execution:Even the final results points to the same object:
Removing the assignment of the return value to
pres
produces the same output.Joshua Yoerger
11,206 PointsJoshua Yoerger
11,206 PointsThat makes sense. Thank you Chris!