Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial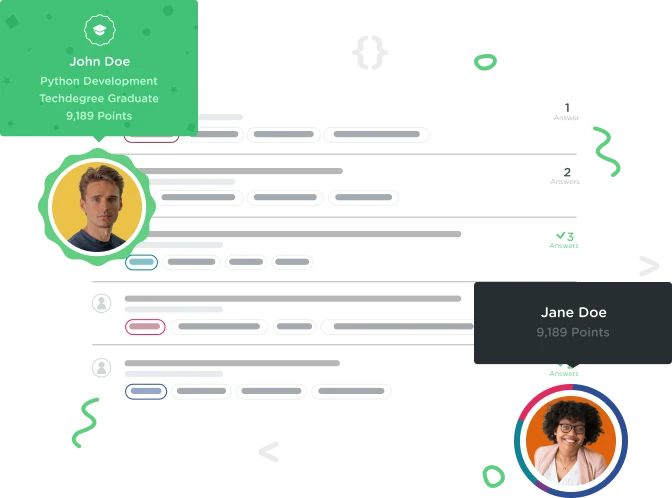
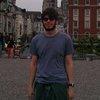
Robert Stefanic
35,170 PointsSkipping items while building a set
So I'm building this set from all of the courses 'prereqs' in this list, but it's saying that my list isn't correct.
I think that the reason my set's incorrect is because I'm including the first title within the courses list, which isn't a prereq for itself -- makes sense. When I print my list locally, I get every title in course including the first one, "Django Basics".
But I'm not sure how to skip the first "title" within the courses list. I need need to have the pres.add(data['title']) statement so I can add the current title before moving onto the next one in the courses dict, but if I move it into my for-in loop, I'll end up only adding the prereqs from the first element in the list.
Any ideas or suggestions? I'm trying to think of another way to build this list, for to change my for-in loop, but all of the ways I've tinkered with it aren't quite right. I either leave out certain prereqs, or add the first title again.
Also, as a side question: does python not allow you to access a key in a dictionary through dot notation? I get an error with "data.title", but "data['title']" is fine. I could have swore that I've used the former before in python, but I could be wrong.
courses = {'count': 2,
'title': 'Django Basics',
'prereqs': [{'count': 3,
'title': 'Object-Oriented Python',
'prereqs': [{'count': 1,
'title': 'Python Collections',
'prereqs': [{'count':0,
'title': 'Python Basics',
'prereqs': []}]},
{'count': 0,
'title': 'Python Basics',
'prereqs': []},
{'count': 0,
'title': 'Setting Up a Local Python Environment',
'prereqs': []}]},
{'count': 0,
'title': 'Flask Basics',
'prereqs': []}]}
def prereqs(data, pres=None):
pres = pres or set()
while data:
pres.add(data['title'])
for x in data['prereqs']:
prereqs(x, pres)
return pres
1 Answer
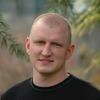
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsSo this one is a bit tricky, you have to really read, look at the data given and think about the desired result.
Now courses really contains just one main course with a subset of courses in it. In normal words we want to say: "I want to see the prereqs of Django Basics.". So I should be able to pass the contents of Django Basics which would be the dictionary key prereqs into my recursive function and have it return me a set of the child courses. But we dont want Django Basics included. This would ideally be a reusable function that we could pass any course a list of prereqs.
Now breaking down your function
def prereqs(data, pres=None):
pres = pres or set()
while data:
pres.add(data['title'])
for x in data['prereqs']:
prereqs(x, pres)
return pres
when we recurse over something its KIND OF like a loop. So be careful when using loops inside loops with recursion. It can get really hairy in there. To pass this you really only need to use 1 loop. a for loop.
A for loop KNOWS how to move on to the next item.
HINT: You only ever want to loop over prereqs for the sole purpose of getting the Title to add to the pres set. BUT you have to ensure once you add you call your recursive function and pass it the updated list so it can repeat that logic until it cannot anymore. :)
Let me know if this helps, as I am trying to guide you to answer without giving you the answer.