Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial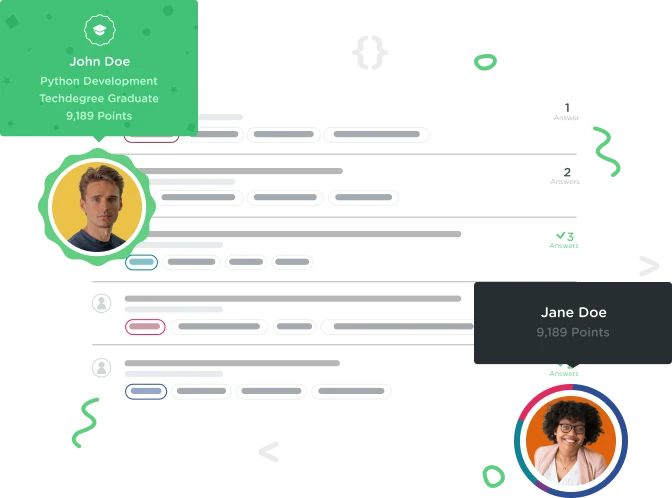

Yao Liu
1,733 Pointsso I've been trying to do this code in IntelliJ IDEA, and it gives me errors. Should it be working?
import java.io.Console;
public class Main {
public static void main(String[] args) {
Console console = System.console();
String name = console.readLine("What is your name?");
console.printf(name);
}
}
that is the code I am trying, its not working on IntelliJ IDEA. Someone help?
3 Answers

Seth Kroger
56,413 PointsEclipse and IntelliJ have issues with java.io.Console. You need to use System.in and System.out instead. System.out works virtually the same as Console for output but for input you need to open a BufferedReader first:
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
// ^^- -If these are red, IDEA can import them for you --^^
System.out.printf("What is your name? ");
String guess = bufferedReader.readLine();
System.out.printf(name);

Tony Andre Haugen
2,807 PointsOr you can use Scanner - this will give you less code to write;
Scanner sc = new Scanner(System.in);
System.out.printf("What is your name? ");
String name = sc.nextLine();
System.out.printf("Hello, my name is %s.\n", name);
System.out.printf("%s is learning how to write Java again.\n", name);
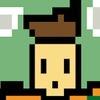
Greenie 99
1,715 PointsThis does not work, as Eclipse and IntelliJ use javaw.exe and not java.exe. To solve your issue, if you want to keep the code that the guy in the video does, just open up a Terminal/Command Prompt window.
Now, find the folder/file that your code is in, and type cd <drag the folder/file in>. Type javac ______.java Type java <class name>