Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial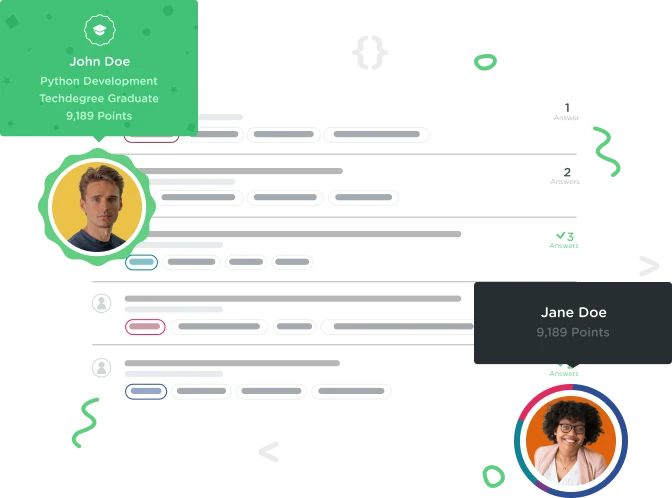

Allan Blain
2,418 Points[SOLVED] C# Basic Task 2 working but throwing this error "System.ArgumentNullException: Value cannot be null"
using System;
namespace Treeshouse.CodeChallenges {
class Program { static void Main() {
int i = 0;
while (true)
{
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
try
{
var n = int.Parse(Console.ReadLine());
while (i < n)
{
Console.WriteLine("Yay!");
i++;
}
break;
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
} } }
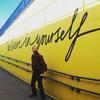
Matthew Hill
7,799 PointsIf you change your code to catch all exceptions does this work? If so then the FormatException is too specific and is missing out on a possible error being generated by the call to int.Parse() function
4 Answers

Allan Blain
2,418 PointsI found and tried this and it worked not exactly sure why the only major difference I could find was the Console.ReadLine at the end.
using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() {
var counter = 0;
Console.Write("Enter the number of times to print \"Yay!\": ");
var input = Console.ReadLine();
try
{
var times = int.Parse(input);
while (counter < times)
{
Console.Write("Yay!");
counter += 1;
}
}
catch (FormatException)
{
Console.WriteLine("you must enter a whole number");
}
catch (ArgumentNullException)
{
Console.WriteLine("You must enter a positive number");
continue;
}
Console.ReadLine();
}
}
}

Tom Ku
3,049 PointsThe problem was Treehouse's automated code checker somehow enters a null value when it tests the program, on the line "var input = Console.ReadLine();" It is a code bug in Treehouse's code checker.
By adding the catch(ArgumentNullException), it catches this mistake.

Dov Brodkin
2,695 PointsPlace the decleration of the variable i IN THE TRY CATCH function. I had the same issue as you and putting the variable in the try catch worked.

Allan Blain
2,418 PointsI tried both suggestions, after adding both catches if still fails but with no error message
while (true)
{
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
try
{
int i = 0;
var n = int.Parse(Console.ReadLine());
while (i < n)
{
Console.WriteLine("Yay!");
i++;
}
break;
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
catch (ArgumentNullException)
{
Console.WriteLine("Can not be left blank.");
continue;
}
}
} } }

Dov Brodkin
2,695 PointsYou don't need int i (just use zero), get rid of break, it currently ends the program too early, you also can't have to catches for one try, look it up on google. When you print Yay! to the screen change the variable to n and zero(look at my code) and use n--; try this code:
while (true){ try { //int i = 0; you don't need int i just use zero Console.WriteLine("Enter the number of times to print \"Yay!\": ");
var n = int.Parse(Console.ReadLine());
while (n > 0)
{
Console.WriteLine("Yay!");
n--;
//break; break just ends the program earlier
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
Hope this helps, please let me know if it works. Good Luck!
Dov Brodkin
2,695 PointsDov Brodkin
2,695 PointsYou should upload your code, so people can see the problem with your example to help you