Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial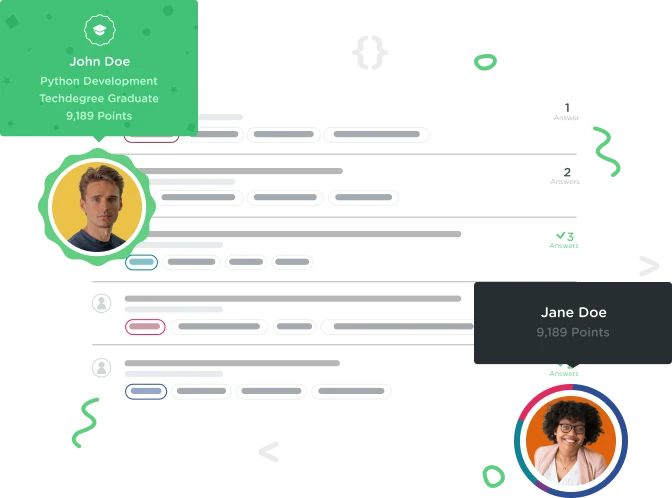

Christer Monsen
3,664 Points[Solved] Can't seem to figure this one out and don't find solution to challenge 2 of 2
How to make this right?
Because I cant seem to understand whats wrong with this.
namespace Treehouse.CodeChallenges
{
class Frog
{
private GetNumFliesEaten _numFliesEaten;
public int GetNumFliesEaten GetValue()
{
return _numFliesEaten;
}
public void SetNumFliesEaten(GetNumFliesEaten value)
{
_numFliesEaten = value;
}
}
}
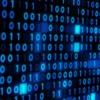
Alexander Davison
65,469 PointsCan you give me a link to the code challenge?

Christer Monsen
3,664 PointsTask 2 of 2. (Accessor Methods)
Task: Write a public getter method named GetNumFliesEaten and a public setter method named SetNumFliesEaten for _numFliesEaten.
https://teamtreehouse.com/library/c-objects/encapsulation-with-properties/accessor-methods-2
6 Answers
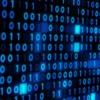
Alexander Davison
65,469 Pointsnamespace Treehouse.CodeChallenges
{
class Frog
{
private GetNumFliesEaten _numFliesEaten;
public int GetNumFliesEaten GetValue()
{
return _numFliesEaten;
}
public void SetNumFliesEaten(GetNumFliesEaten value)
{
_numFliesEaten = value;
}
}
}
This is your code. It look really neat! Two problems, though. One, there's no use to say GetValue() in "public int GetNumFliesEaten GetValue()" . Try getting rid of that GetValue(). Second, you called the parameter for SetNumFliesEaten value, which is a reserved keyword in C#. "value" is like a built-in reserved word in C#, just like how "public" and "void" are. You cannot use them as variable names, or it will cause an error. instead, try giving it a different name that isn't a reserved word. I'm going to use "val" as the new name, but you can call it whatever you want. Otherwise, I really like your code!
EDIT: Also, val's type should be a int, not a GetNumFliesEaten!
Here's the solution (please read the text above this first, please please! ):
namespace Treehouse.CodeChallenges
{
class Frog
{
private GetNumFliesEaten _numFliesEaten;
public int GetNumFliesEaten()
{
return _numFliesEaten;
}
public void SetNumFliesEaten(int val)
{
_numFliesEaten = val;
}
}
}
Hope this helps! ~Alex ;smile:
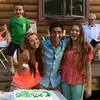
David Axelrod
36,073 PointsSeems to me that value is a contextual keyword :( https://msdn.microsoft.com/en-us/library/x53a06bb.aspx
Try a different variable name. Otherwise your code looks sharp (hehehe) to me!

Christer Monsen
3,664 PointsThanks very much Alex, but I get message that it will not compile for some odd reason. And thanks that you like my code :) Really appreciated.
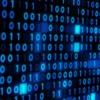
Alexander Davison
65,469 PointsNo problem :) What's the message? I can help you.

Christer Monsen
3,664 PointsBummer! Your code could not be compiled. Please click on "Preview" to view the compiler errors.
Frog.cs(5,17): error CS0246: The type or namespace name `GetNumFliesEaten' could not be found. Are you missing an assembly reference? Compilation failed: 1 error(s), 0 warnings
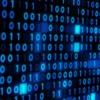
Alexander Davison
65,469 PointsCan you press Preview And tell me what that says? Thx

Christer Monsen
3,664 PointsGot it! Here is what worked for me :)
namespace Treehouse.CodeChallenges { public class Frog { private int _numFliesEaten;
public int GetNumFliesEaten()
{
return _numFliesEaten;
}
public void SetNumFliesEaten(int value)
{
_numFliesEaten = value;
}
}
}
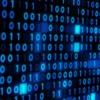
Alexander Davison
65,469 PointsCool! I'm new to C# and I didn't know that you could use value as a parameter. I though is would cause an error :)
Thanks a ton! ~Alex

Layth Eshuwaili
12,128 PointsI can't make my code to work. Any suggestion?
namespace Treehouse.CodeChallenges { class Frog { private int _numFliesEaten;
public int GetNumFliesEaten()
{
return _numFilesEaten;
}
public void SetNumFliesEaten(int x)
{
_numFliesEaten = x;
}
} }

Layth Eshuwaili
12,128 PointsNever mind, it worked.
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsHi Christer Monsen,
Which challenge is this for?