Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial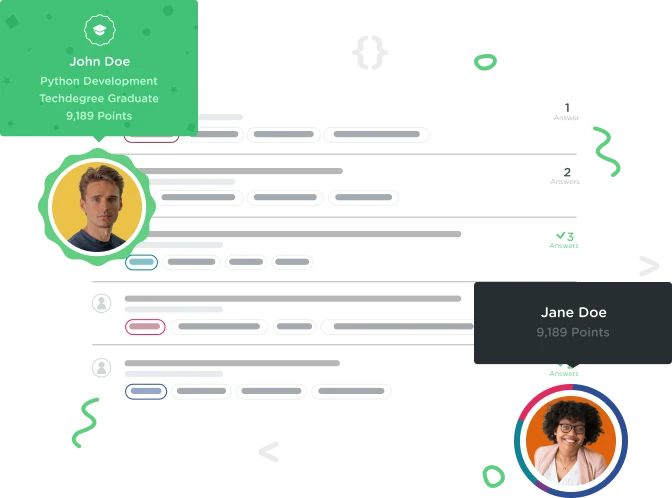

taejooncho
Courses Plus Student 3,923 PointsStuck in Capitalism, the Game part 3
Hello, I think I am trying to do what the task is asking me to do, but I can't seem to find out where in my code I am doing wrong to fix the issue.
I have rewatched the lecture video that talks about classmethods, and I still feel lost. Would anyone please guild me and explain what I am doing wrong.
Thank you in advance.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size = 2, die_class = D6)
@property
def doubles(self):
return self[0] == self[1]
@classmethod
def reroll(cls):
if self[0] == self[1]:
return cls().__init__()
else:
return cls(self)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
2 Answers
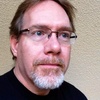
Chris Freeman
Treehouse Moderator 68,441 PointsYou are very close. The method reroll
does not need to recheck if doubles are present.
remove if statement
the second return statement is all that's needed, but without the
self
argument
Edited: The correct return statement should be return cls()
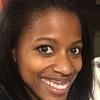
nicole lumpkin
Courses Plus Student 5,328 PointsYikes, I've gone over this entire section twice including the Constructicons vid on classmethods and I still do not understand why the answer is simply
return cls().__init__()
I'm not even familiar with this syntax. I've only seen a return with cls look something like this:
return cls(something_in_here)
Any additional information would be great, I'm so lost on this one
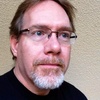
Chris Freeman
Treehouse Moderator 68,441 PointsThank you nicole lumpkin!! You post a most excellent question. I've dug deeper and chatted with Kenneth Love about this challenge. The for cls().__init__()
does not make sense it effectively runs __init__()
twice, once on instance creation and again on the explicit call to __init__()
. However, since __init__()
does not return anything, None
would be returned from the @classmethod
.
So the correct answer in this case should have been
return cls()
Until today, this challenge checker accepted both answers as correct. Now only the above answer will work. I've updated my answers on this thread to reflect this change.
Post back if you have more questions. And Thanks Again!!!
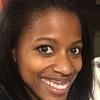
nicole lumpkin
Courses Plus Student 5,328 PointsThank you so much Chris Freeman!! You're help is so appreciated! It can be difficult learning this on your own, plugging away day after day. Thanks for making me and I'm sure many others feel valued and looked after in the Community.
taejooncho
Courses Plus Student 3,923 Pointstaejooncho
Courses Plus Student 3,923 PointsHello Mr.Freeman, As always thank you for all your help, it has really helped me tremendously!
I saw your suggestion, but may I ask why I need to remove if statement?
My interpretation of the task was that if I have doubles I would return a new instance. If I remove the if statement how can I make the code so that Python will reroll if there is double..
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsBecause regardless of the
if
results,return cls()
should be the next statement
Edited: to match updated challenge checker.
taejooncho
Courses Plus Student 3,923 Pointstaejooncho
Courses Plus Student 3,923 PointsAh, okay that makes sense. When I implemented your suggestion it solved the challenge.
Can I also ask if this code (return cls(self)) has no errors? Originally, I thought that it was this line that was giving me errors..
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsIn
cls(self)
, theself
is not defined in a classmethod.