Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial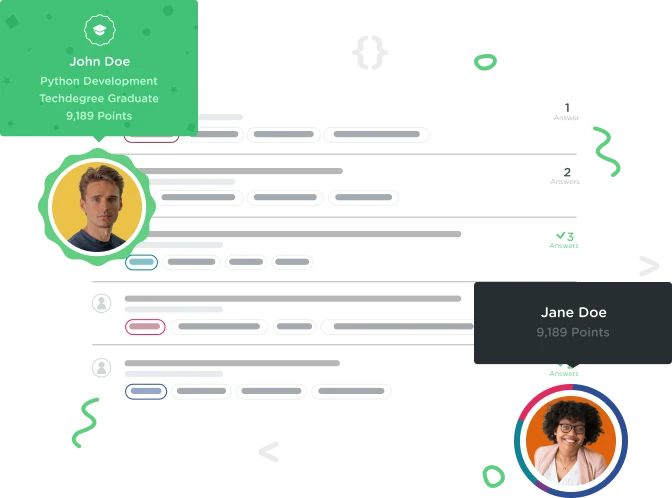

Eric Tang
Courses Plus Student 1,300 PointsStuck on questions
I am so frustrated. Sometimes it really confuses me because each line is not explaining in detail what they do so I never fully understand. And it confuses me even further when the practice is different. I tried this code but it keeps saying that I need to add a new method names getCount with char letter in its parameter. I did it but still doesnt work. Help!
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public String getCountOfLetter(char letter){
int count=0;
for(char letter: tiles.toCharArray()){
if(tile.indexOf(letter)){
count=letter;
}
}
return count;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
6 Answers
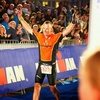
Steve Hunter
57,712 PointsHi Eric,
The first part of this question asks: Create a new method named getCountOfLetter that returns an int, and requires a parameter of type char named letter. For this task, just make it return 0.
Let's do that step by step. First, the method is called getCountOfLetter()
- it takes a char
called letter
as a parameter and returns an int
(not a String). Let's assume it is public
and make it return zero inside the method body.
That looks like:
public int getCountOfLetter(char letter){
return 0;
}
Next you want to do pretty much what you have done. You've set up a for
loop and iterated over tiles
. Inside the loop, check if the loop variable (I called mine l
) is equal to the char
passed in as a parameter(letter
). If they are equal, increment a counter. After the loop, return the value of that counter variable.
That could all look like:
public int getCountOfLetter(char letter){
int count = 0;
for(char l : tiles.toCharArray()){
if(l == letter){
count++;
}
}
return count;
}
Let me know if you need more assistance.
Steve.
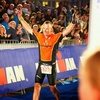
Steve Hunter
57,712 PointsHi Eric,
Glad you got it fixed! Sorry if my initial response wasn't clear enough.
So, here you're asking what indexOf()
is doing. This method returns the position in the string of the parameter. So, if letter
is the second letter in the hits
string, the indexOf()
method will return 1 (arrays start counting at zero, so 1 is the second element's index). If letter
is not in hits
then the indexOf()
method returns -1.
So, Craig is testing if hits.indexOf(letter) > -1
which tells you that letter
is contained in hits
.
If hits
contains T R E E H O U S E
, then:
hits.indexOf('H'); // returns 4 - greater than -1
hits.indexOf('S'); // returns 7 - greater than -1
hits.indexOf('Z'); // returns -1
Make sense?
Steve.
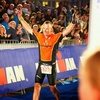
Steve Hunter
57,712 PointsHi Eric,
Apologies for the slow response; I headed out for a run (and got snowed on!).
I'm not sure where answer.indexOf()
is used. Is there a workspace for this course?
Looking at the code you've asked about, you have a method that must be part of a class which has member variables called hits
and answer
else those variables won't exist. In this method, you are iterating over answer
by converting the String
to an array using toCharArray()
. At each iteration, you are using a char
to identify whether each letter is contained within hits
. I don't really understand how the progress
variable is going to work out.
I'm assuming it's supposed to build up steadily as each letter is correctly guessed; something like:
_ _ _ _ _ _ _ _ _
Guess a T
gives:
T _ _ _ _ _ _ _ _
Guess an E
and you get:
T _ E E _ _ _ _ E
And so on. I'm not sure that's what the code does. But I'd need to see how the rest of it is implemented.
Sorry that doesn't help much. But without knowing what hits
and answer
are and how they are created & updated, I can't answer about the distinction between them. I'll see if I can get hold of the code ...
Steve.
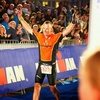
Steve Hunter
57,712 PointsYes, it does populate the progress
variable with letters or dashes depending whether that particular letter has been guessed or not.
So, each "hit" is stored in a random string of letters in the order of correct guesses made. That's the variable hits
. The for
loop here iterates through the answer
variable (which contains "treehouse" - lower case & case sensitive). At each pass, we test to see if each letter of t r e e h o u s e
is contained within hits
. That's what indexOf()
does if you compare the returned value to -1. If the returned value is NOT -1, then the character is contained within the string. If the returned value is -1, then the character is NOT in the string.
If this is that case, the letter is revealed as the loop goes over each character in the answer
variable in turn. So every letter in the answer is tested to see if it is part of the hits
dtring. If it is, that letter is revealed, if it is not, the letter is left as the dash symbol.
Make sense?
Steve.

Eric Tang
Courses Plus Student 1,300 PointsI finally get it know. Thank you. If you don;t mind. In the video, where Craig was typing out the code
public String trackProgress(){
String progress="";
for(char letter: answer.toCharArray()){
char display='_';
if(hits.indexOf(letter)>-1){
display=letter;
} progress+=display;
}return progress;
}
Could please explain to me what is actually going on after the for statement. I'm confused as to why hits.indexOf(letter) is there. I just can't wrap my head around what it's saying.

Eric Tang
Courses Plus Student 1,300 PointsIt starting to make sense now, But i;m still a little confused. What I want to know is what is the difference between answer.indexOf(letter) vs hits.indexOf(letter). The first one is saying finding out the position of 'letter' in the whole answer which makes sense with the name of the method. But the second one is saying finding out the position of letter in hits?? How does that work? Letter in the latest code I posted is pretty much the answer spread out into char. And hits is pretty much the letter we enter in right? So is the second one saying, which position of answer is in letter?

Eric Tang
Courses Plus Student 1,300 PointsAhhh I get it now. I was confused before as in Craigs tutorial video he uses answer.indexOf(letter) . So I thought the beginning of this code has to contain more characters as it's trying to find one char in a group of many chars. So I thought that hits.indexOf(letter) wouldn't work. Thank you so much. I would've been stuck forever without your help
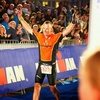
Steve Hunter
57,712 PointsNo problem; glad to help!