Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial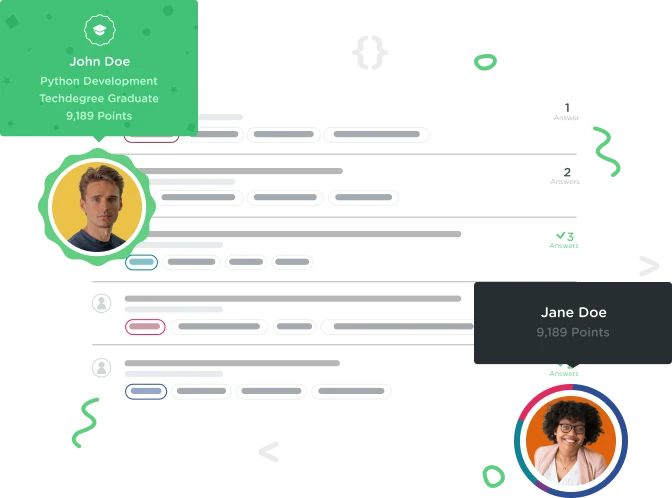

Hazel Dhliwayo
4,321 PointsSTUCK WITH A CODE CHALLENGE
I don't know whats wrong with my answer, my friend tried it on her workspace and it worked
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, laps, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = laps
for key, values in kwargs.items():
setattr(self, key, value)
self.laps = laps
def run_lap(self, length):
self.laps += 1
self.fuel_remaining -= length *0.125
2 Answers
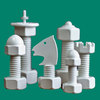
Steven Parker
231,248 PointsThe workspaces aren't always the best way to work on challenges, since they don't provide test data nor do they enforce any performance criteria. It's easy to get a "false positive" if you misinterpret the objective.
I see two issues at first glance:
- the "kwargs" loop iterates "values" (plural), but the
settattr
is referencing "value" (singular) - "laps" is maintained internally but should not be a required argument to create an instance
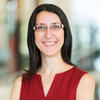
Louise St. Germain
19,424 PointsHi Hazel,
I will echo what Steven said, plus a couple more things, now that I've played with your code to figure out why it's not running.
Here are the things that will need fixing (they are all minor), some of which Steven already mentioned but I'll repeat them here to make a complete list:
- You will need to make sure that when you loop through "key" and "value" for **kwargs, you use exactly the same spelling in subsequent code. So where you have "values" in the for loop, and "value" later in the code, Python thinks these are two completely different variables. To fix this, take the "s" off "values" in the for loop:
for key, value in kwargs.items():
setattr(self, key, value)
- You have a mix of spaces and tabs in your code (which Python is really sensitive to), and you also have different indentation for the "def __init__" line (= 5 spaces) than for "def run_lap" (= 1 tab). "def run_lap" specifically has a problem because you have a tab in front of it, and even when it's converted to (4) spaces, it still doesn't line up with the other method definition, which triggers an error. You can fix this problem by converting the tab to spaces, and making sure that these two methods have the same number of spaces in front of them:
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, laps, **kwargs):
# ... etc ...
# Convert the tab to a space here, and line up the two def's so they are
# exactly in line with each other
def run_lap(self, length):
self.laps += 1
self.fuel_remaining -= length *0.125
You can delete the second self.laps = laps line, first because it's a duplicate, and also because it has that same tab problem.
To avoid this mixed tabs/spaces problem in the future, make sure your workspace is set up so that it converts tabs to spaces (you will see "spaces" or "tabs" at the bottom right hand corner - if you use "spaces" and "4", this setting will match the Challenge set up, which will prevent this type of problem from happening.
Like Steven said, you should remove "laps" from the arguments for the __init__ method - it's only set internally, not by the user. (One less thing for them to worry about! :-))
Finally (almost there! :-)), the last part of the challenge asks you to set laps to 0 within the __init__ method, so you'll need to change self.laps = laps to self.laps = 0. (You don't really need that first "laps = 0" right after the "class RaceCar:" line, although it doesn't hurt.)
In the end, your adjusted code will look like this:
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.laps = 0
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length):
self.laps += 1
self.fuel_remaining -= length *0.125
Good luck! I hope this helps, and let me know if you still have any questions.
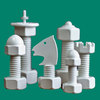
Steven Parker
231,248 PointsGood catch on the indentation, I guess need I my eyes recalibrated.
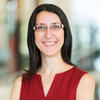
Louise St. Germain
19,424 PointsHaha! I think we can both go in for calibration then - I didn't notice it either until my code linter picked it up! :-)

Hazel Dhliwayo
4,321 PointsThank you very much, it worked