Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial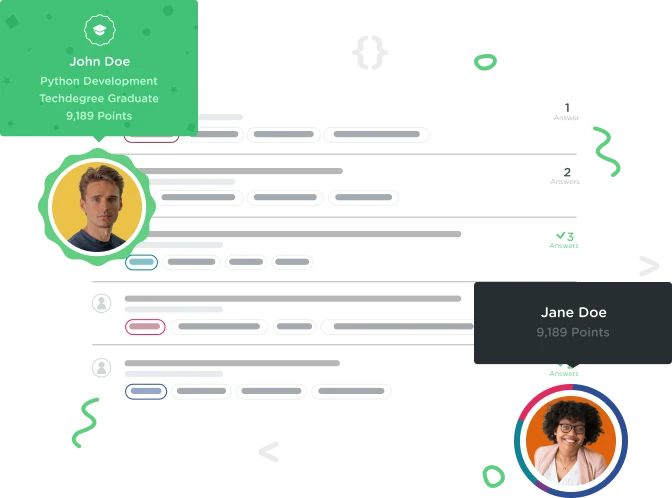
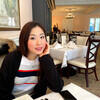
Hanwen Zhang
20,084 PointsTake a minute to review the files in this challenge. For this task, you'll need to add plants to the database. Inside of
Take a minute to review the files in this challenge. For this task, you'll need to add plants to the database. Inside of app.py, you have a form route and function. This is where you will add your submitted plants to your database. Inside of the given if statement (before the return redirect()), you need to create a Plant object using the Plant() model and save it to a varaible called new_plant. Then add the plant to the database with db.add() and commit it with db.commit().
from models import db, Plant, app
from flask import render_template, url_for, request, redirect
@app.route('/')
def index():
return render_template('index.html')
@app.route('/form', methods=['GET', 'POST'])
def form():
if request.form:
new_plant = Plant (type=request.form['type'],
status=request.form['status'])
db.add(new_plant)
db.commit()
return redirect(url_for('index'))
return render_template('form.html')
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///plants.db'
db = SQLAlchemy(app)
class Plant(db.Model):
id = db.Column(db.Integer, primary_key=True)
plant_type = db.Column(db.String())
plant_status = db.Column(db.String())
{% extends 'layout.html' %}
{% block content %}
<form action="{{ url_form('form') }}" method="POST">
<label for="type">Plant Type:</label>
<input type="text" name="type" id="type" placeholder="Plant type">
<p>Plant Status:</p>
<label for="good">GOOD</label>
<input type="radio" name="status" id="good">
<label for="ok">OK</label>
<input type="radio" name="status" id="ok">
<label for="bad">BAD</label>
<input type="radio" name="status" id="bad">
</form>
{% endblock %}
{% extends 'layout.html' %}
{% block content %}
<h1>Our Plants:</h1>
<div>
<h2>Plant Type</h2>
<p>Plant Status</p>
</div>
{% endblock %}
1 Answer
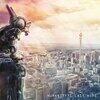
Trenton Spears
Python Development Techdegree Graduate 16,969 PointsHey Hanwen Zhang!
I had the same problem as well. So one thing I noticed.
1) Inside of the "if statement" in your def form()
function I believe you're misreferring both objects inside of the Plant()
model in order to properly assign your new variable new_plant
as they should be specified directly in reference. Simply put, you should change both of your objects from type
and status
to plant_type
and plant_status
(refer to models.py
class method).
Overall, I think your app.py
file should look something like this..
from models import db, Plant, app
from flask import render_template, url_for, request, redirect
@app.route('/')
def index():
return render_template('index.html')
@app.route('/form', methods=['GET', 'POST'])
def form():
if request.form:
new_plant = Plant(plant_type=request.form['type'],
plant_status=request.form['status'])
db.add(new_plant)
db.commit()
return redirect(url_for('index'))
return render_template('form.html')
@app.route('/delete')
def delete():
return redirect(url_for('index'))
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)