Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial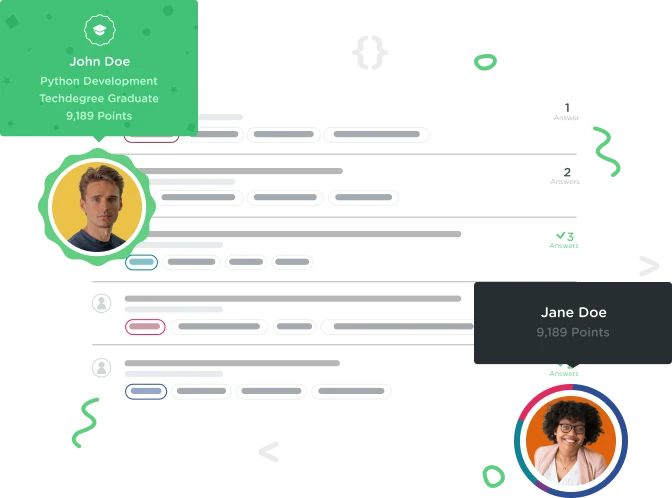

Isak Landström
2,882 PointsThe application - lists with Craig
Hello!
I challenged myself of doing the application of the shopping list before watching any of the videos of how Craig made it, please take a look at my code and I would love come constructive criticism on what I am doing that is good and what I can improve on. Here is my take on the shopping list application:
print("Welcome to the shopping app\n" +
"Here you will be able to write down your shopping list\n" +
"for help: just write 'HELP'")
# Define a help function
def show_help():
print("\n* To add an item just write what you want to add" +
"\n* For help, just write HELP" +
"\n* To show the shopping list, write: Show" +
"\n* To remove an item from the list, write: Remove" +
"\n* To finish writing your shopping list, write: Done" +
"\n* To clear shopping list, write: Clear"
)
# Define add to list function
def add_to_list(item):
print(item.lower(), "added to the list")
shopping_list.append(item)
# Define show list function
def show_list():
noun_ending = "s"
if len(shopping_list) < 2:
noun_ending = ""
print(f"\nYour shopping list cointains of {len(shopping_list)} item{noun_ending}\n")
for idx, item in enumerate(shopping_list, 1):
print(f"{idx}.", item.lower())
# Define remove function
def remove_from_list(item_to_remove):
try:
shopping_list.remove(item_to_remove.upper())
except ValueError:
try:
item_to_remove = int(item_to_remove)
except ValueError:
print("Sorry, that item does not exist in your shopping list")
else:
if item_to_remove > 0 and item_to_remove <= len(shopping_list):
print("You removed {} from the shopping list".format(shopping_list[item_to_remove - 1]))
shopping_list.pop(item_to_remove - 1)
else:
print("Sorry, that item does not exist in your shopping list")
else:
print("You removed {} from the shopping list".format(item_to_remove))
shopping_list = []
loop_valid = True
while loop_valid:
user_choice = input("\nWhat would you like to add to the list? ").upper()
try:
user_choice = int(user_choice)
except ValueError:
if user_choice == "HELP":
show_help()
elif user_choice == "SHOW":
if len(shopping_list) > 0:
show_list()
else:
print("Your shopping list is currently empty")
elif user_choice == "REMOVE":
show_list()
remove_item = input("\nTo remove an item, please specify index position or item by value:")
remove_from_list(remove_item)
elif user_choice == "DONE":
print("This is your shopping list, happy shopping!")
show_list()
loop_valid = False
elif user_choice == "CLEAR":
shopping_list.clear()
print("Your shopping list is now cleared")
else:
add_to_list(user_choice)
2 Answers
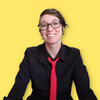
Mel Rumsey
Treehouse ModeratorWow, awesome job on this Isak Landström!
I went through your program and it seems to be working flawlessly. Nothing I enter causes it to crash so there is some solid error handling here. Your functions and variable names are descriptive and your code is tidy and easy to follow.
Great job on this! 💪💪💪 Keep up the awesome work :D
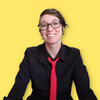
Mel Rumsey
Treehouse ModeratorLooking forward to seeing your work!! 🙌
Isak Landström
2,882 PointsIsak Landström
2,882 PointsHello!
Thank you very much for the feedback, really a boost to my confidence! I will probably be posting more with my upcoming challenges with Python :)