Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial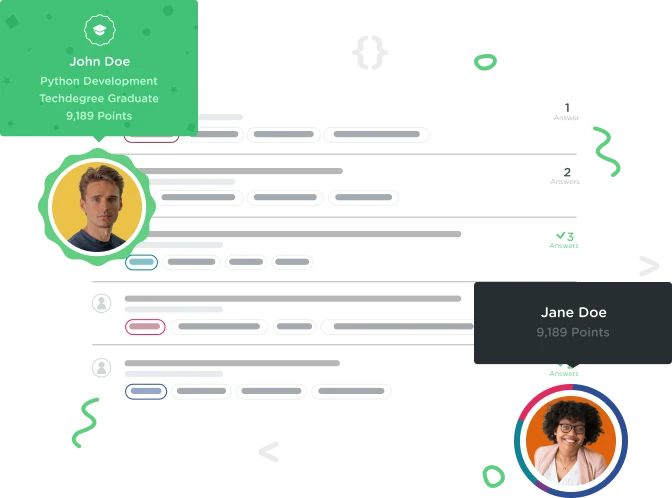
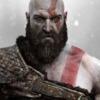
boi
14,242 PointsThe "yield" keyword and understanding its use in a code challenge. Python OOP
Hello, I am having trouble understanding the use of "yield". What I understand so far about yield
is that it works similar to return
but return
ends a function while yield
produces output while running.
I read a post in the TreeHouse Community where it explained and I quote
"After a "return", if a function is called again it will start over from the beginning. But after a "yield", the next time it is called it will continue from where it left off."
I understand this concept but I can't seem to make use of it practically, I completed a code challenge where I had to define an __iter__
method which used the yield
in it, I successfully completed the challenge but I copy/pasted the code of the challenge to see it's working and frankly, I don't see why yield
is even used here or the __iter__
method altogether.
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
def __iter__(self):
yield from self.cells
class TicTacToe(Board):
def __init__(self):
super().__init__(3,3)
I tried experimenting with the above code ( which is the challenge code ) to understand the use of yield
in the python REPL (below)
>>> game = TicTacToe()
>>> game.cells
>>> #output [(0, 0), (1, 0), (2, 0), (0, 1), (1, 1), (2, 1), (0, 2), (1, 2), (2, 2)]
# comment ( the above output could be achieved without the use of yield or __iter__)
# so what exactly is the purpose of yield in this code?
Conclusion:
All I want to know is what happened when I used the yield
function in the code above, as I can't seem to make use of the yield
function in the REPL, I would really be grateful if someone could give me a practical example instead of theory
2 Answers

Myers Carpenter
6,421 Pointsboi Let's say you want to read thru the contents of a file, but it's a big file, so you don't want to load it all into memory. Handling this is built in to python, but reimplementing it might help understand yield
:
def read_chunk(filename, chunk_size):
with open("big-file.txt", "r") as ff:
while True:
buffer = ff.read(chunk_size)
if buffer == '': # if we've reached the end of the file
break
yield buffer
for chunk in read_chunk('bigfile.txt', 1024):
print(chunk)
Here we read the whole file, but we never have more than 1024 bytes of it in memory.

Myers Carpenter
6,421 PointsFunctions that use yield
are called "generators".
Generators are useful for being "lazy": only compute an answer when you need it. For example range(1000)
doesn't just return a list with 1000 numbers, but uses yield
to return only the next number when it's time. Maybe you'll need all 1000 numbers, maybe you'll break out of the loop before you get finished.
Now say that you did a select from a database that returned 1 million rows and started working thru them. It would be inefficient to if in all cases you fetched all 1 million rows from the database, so we use a generator that fetches a batch fo 100 rows at a time.
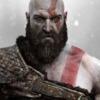
boi
14,242 PointsThx for your answer, I did understand your answer, Is it possible if you could give me a code example, like anything practical?