Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial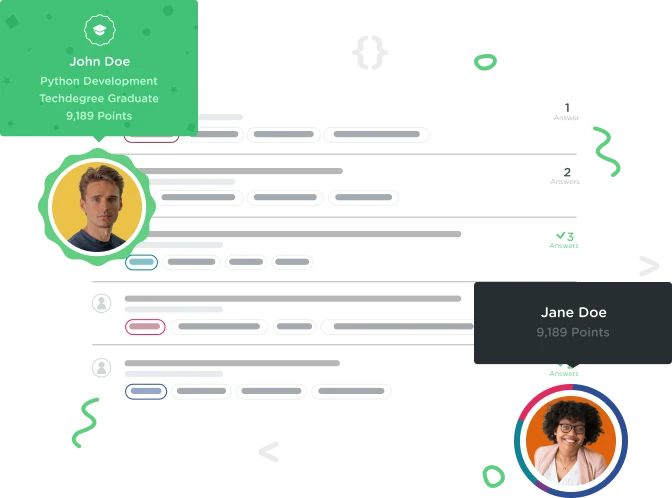

Y B
14,136 Pointstimemachine question
I get that an error that the format doesn't match.
Also what is the int for in this question?
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
def time_machine(int1, strdate):
tdelta = datetime.datetime.strptime(strdate, '%M %H %d %Y')
new_time = starter + tdelta
8 Answers
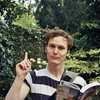
Bart Bruneel
27,212 PointsThe integer is the number of years, days, hours or seconds you give in. So, the input is for example integer=5 string=years. You'll first need to switch years to days by multiplying them with 365 and changing the string to days. Then you have to write several if and elif statements for if the input string is equal to days, hours or seconds. For example if the input string is equal to years, you first switch it to days and the resulting time delta needs to be added to starter, as in starter+datetime.timedelta(days=5*365). I have tried putting the input string into the time delta method but it needs to be first changed to a keyword. I have no idea how to do that.
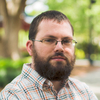
Kenneth Love
Treehouse Guest TeacherI added an example and changed the prompt some to hopefully make it more obvious for future students.
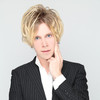
Mikael Enarsson
7,056 PointsThis is not the right direction at all, but I don't blame you since the question was very oblique in what it wanted you to accomplish.
What they want you to is to write a function that takes two parameters, an int and a string ("minutes", "hours", "days", or "years"). If the string is minutes, return a datetime object that is int minutes in the future from starter, if it is hours, return a datetime that is int hours in the future.
I hope this helps!

Y B
14,136 Pointsah ok I thought the string would be a combine 'YY DD HH SS' etc... thanks
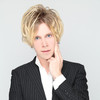
Mikael Enarsson
7,056 PointsYeah, so did I at first ^^'

Y B
14,136 Pointshmm well this didn't work... why doesn't treehouse print the input it's testing with and the output like udacity - it makes it a nightmare to know where the issue is?
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
def time_machine(int1, strdate):
if strdate == 'minutes':
tdelta = datetime.timedelta(hours=int1)
elif strdate == 'hours':
tdelta = datetime.timedelta(hours=int1)
elif strdate == 'days':
tdelta = datetime.timedelta(days=int1)
elif strdate == 'years':
tdelta = datetime.timedelta(days=int1*365)
new_time = starter + tdelta
return new_time
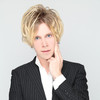
Mikael Enarsson
7,056 PointsYou made a mistake inside the first if, increasing hours instead of minutes.
Oh, and I agree, the output would be very nice.

Y B
14,136 PointsThanks for spotting that Mikael.
Kenneth - thanks yes a better prompt would definitely help.
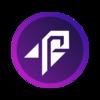
Rocket Dollar Invest
7,129 PointsA simple solution for this problem is to first check if the string is 'years' and if so change the string to 'days' since timedelta will not except years as an argument. Then multiply the integer by 365 to get the equivalent number of days for the given number of years. Finally, return the duration as the difference of the starter datetime and the timedelta of the string and integer passed to the method as a literal dictionary with the ** prefix operator to unpack the dictionary.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(integer, string):
if string == 'years':
string = 'days'
integer = integer * 365
return starter + datetime.timedelta(**{string: integer})