Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial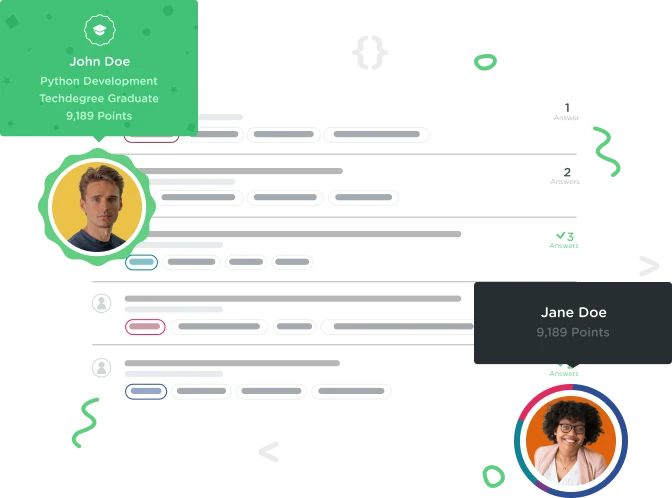

Elizabeth McInerney
3,175 Pointstime_machine timedelta
I am getting "Bummer! Try again" for this code.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(an_integer, a_string):
if a_string == 'years':
a_string = 'days'
an_integer = an_integer*365
return starter + datetime.timedelta('a_string' = an_integer)
6 Answers
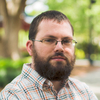
Kenneth Love
Treehouse Guest Teachertimedelta
has no idea what 'a_string'
is. Try it without the quotes. I'm not sure that'll pass, but it's a pretty good solution.

Elizabeth McInerney
3,175 PointsI have tried it with and without quotes there. I meant to copy the code without quotes. Sorry about that!
Without quotes, it runs in Workspaces, but I get "Bummer try again", in the challenge.
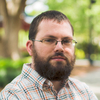
Kenneth Love
Treehouse Guest TeacherHmm, yeah. OK, so, remember in Python Collections when we talked about unpacking a dictionary into keyword arguments for a function call?
Put your a_string
and an_integer
into a dict as key and value, then unpack that dictionary when you call timedelta
.

Elizabeth McInerney
3,175 PointsThat worked, thanks! But why did my previous code work (without the quotes) in Workspaces?
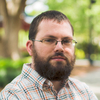
Kenneth Love
Treehouse Guest TeacherI'm not certain. I'll try to debug it and see what I come up with.
Hmm, it errors for me in Workspaces, too:
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(an_integer, a_string):
if a_string == 'years':
a_string = 'days'
an_integer = an_integer*365
return starter + datetime.timedelta(a_string = an_integer)
time_machine(5, 'days')
Generates:
TypeError: 'a_string' is an invalid keyword argument for this function

Elizabeth McInerney
3,175 PointsOk, that's interesting. I can usually find bugs without calling the function. Then I go back to the challenge, and if it worked in Workspaces, it usually works in the challenge. So I did not have your last line above in my code. Without it, the prompt just comes back with no errors. With it, I get the error you listed above.
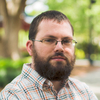
Kenneth Love
Treehouse Guest TeacherAh. Well, without that last line, the function isn't actually being called, so it's valid syntax but you don't know if it actually works or not.

Elizabeth McInerney
3,175 PointsBut I guess I am still confused as to why it doesn't work, because in the Time Deltas video: now+datetime.timedelta(days=3) worked.
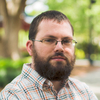
Kenneth Love
Treehouse Guest Teacherdays
is a valid keyword argument for timedelta
, but a_string
isn't.

Elizabeth McInerney
3,175 PointsOk, now we are getting to something that keeps stumping me. But isn't a_string an object that points to the string 'days'? And isn't this just like being a variable that holds 'days'?

Elizabeth McInerney
3,175 PointsOk, now we are getting to something that keeps stumping me. But isn't a_string an object that points to the string 'days'? And isn't this just like being a variable that holds 'days'?
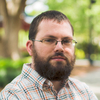
Kenneth Love
Treehouse Guest TeacherLet's make a fake timedelta
function.
def timedelta(microseconds=None, seconds=None, days=None):
return 'timedelta'
Since we've defined exactly what arguments can come in, if we call it with a new argument name, it'll throw an error. Yes, a_string
points to the string 'days'
but that's not being read as an argument/keyword because Python doesn't interpret strings that way. Taking that leap, from a string to an argument name, is something Python only does when we tell it to and, AFAIK, there's no way to tell it to do that with just a string, which is why we put it into something we can unpack, a dict.