Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial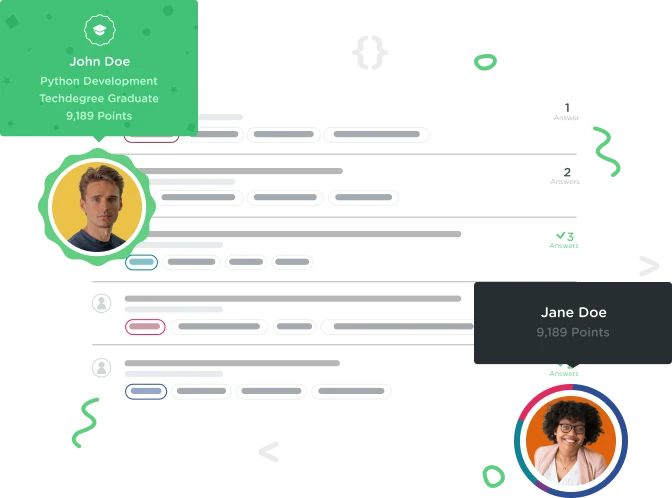
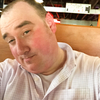
Victor Curtis Jr
5,268 PointsTrouble With TODO's in Python Code
I'm just having trouble with getting this code to work and was wondering if anyone would be willing to help.
#create a new empty list named shopping_list
shopping_list = []
#create a function named add_to_list that declares a parameter named item
#add the item to the list
def add_to_list(item):
item = input("Please enter an item name: ")
shopping_list.insert(item)
def show_help():
print("What should we pick up at the store!")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
""")
show_help()
while True:
new_item = input("> ")
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
add_to_list(new_item)
#call add_to_list with new_item as an argument
3 Answers

jb30
44,806 Pointswhile True:
new_item = input("> ")
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
add_to_list(new_item)
The line add_to_list(new_item)
is not indented, so it is not in your while loop. If you indent it to the same level as your if-else statement, then it should call the add_to_list
function if the input is not 'DONE' or 'HELP'.
def add_to_list(item):
item = input("Please enter an item name: ")
shopping_list.insert(item)
You have passed in item
to add_to_list
, but you are ignoring its value, and asking for more user input. You could change that first line to def add_to_list():
and get the same result. If you want to use the value of item
that is passed to add_to_list
, you could remove the line item = input("Please enter an item name: ")
.
You might want to consider printing out your list or otherwise using it once you are done adding to it.
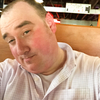
Victor Curtis Jr
5,268 Pointstreehouse:~/workspace$ python -i shopping_list.py
What should we pick up at the store!
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
> milk
> eggs
> butter
> ice cream
> DONE
>>> shopping_list
['milk', 'eggs', 'butter', 'ice cream']
>>> ```
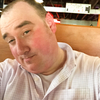
Victor Curtis Jr
5,268 PointsSo I was able to finally get the code to work by troubleshooting. I noticed that the code worked before with DONE
added to the list when add_to_list(new_item)
was called outside the while loop. I took this outside the loop again to see if DONE
would appear in the list again and it did. I used the shopping_list.extend(item)
this time so it added it showing [D' O' N' E']
. So I re-indented the shopping_list.extend(item)
and added just milk
to the list and it added it showing [m' i' l 'k']
. So I changed it to shopping_list.append(item)
and ran the program again and it added [milk]
to the list. So I added some other items and it worked. It was not working before with the same code. I had to unindent and re-indent to get it to work. Is this a bug within python itself? Is this a bug in the shell? Just curious. Because the code was the same before showing and empty list []
and now it fills the list with no change, just the troubleshooting I did.
Victor Curtis Jr
5,268 PointsVictor Curtis Jr
5,268 PointsI definitely understand now about indenting the function call
add_to_list
in the while loop so thatDONE
andHELP
now function correctly. I think I am still having trouble adding items to the list because now when I run the program - no matter how many items I try to add to the list, the list comes out empty[]
.Something seems wrong with this function call: