Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial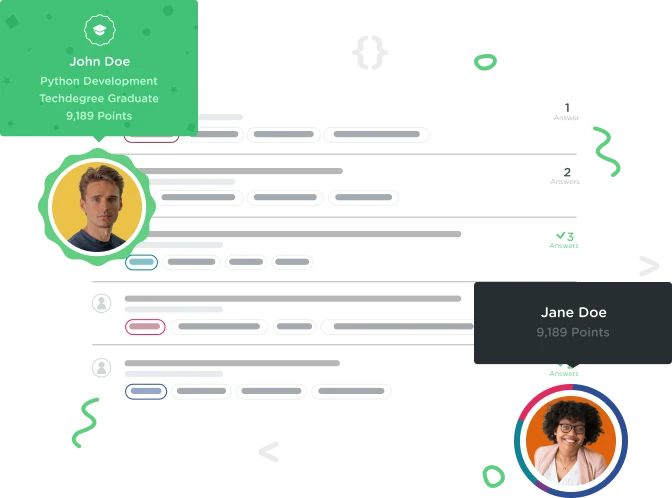

Hina K.
4,806 PointsUnderstanding Classes in Python
class Food:
def __init__(self, meal, spice_level, cost, **kwargs):
self.meal = meal
self.spice_level = spice_level
self.cost = cost
for key, value in kwargs.items():
pass
print("Dinner: {} / Spice Level: {} / Price: ${}. / {}: {}".format(meal, spice_level, cost, key,value))
b1 = Food('Tacos','spicy',4, Cheese='Yes')
b2 = Food('Pizza','not spicy',10, Cheese='No')
Question - I wrote the above Class but I want to make sure i am understanding it correctly..
Class: Food
Instance: b1 = Tacos and b2 = Pizza
Instance variables a/k/a Attributes: meal, spice level, cost, and kwargs (Each instance has its own set of attributes.)
Method: the def function within the class.
Am I interpreting the bolded terms correctly?
Also, does "self" always refers to the first instance? For example, in my code, is the 'self' tacos and pizza?
1 Answer
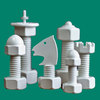
Steven Parker
231,007 PointsThe identifier "self" always refers to the current instance, meaning the one being used or constructed. So when you use b1
, "self" would refer to the object that has "Tacos" as the meal attribute. And when you are using b2
, "self" would be the object that has "Pizza" as the meal attribute.
And yes, your use of the terms seems correct.
Hina K.
4,806 PointsHina K.
4,806 PointsThanks!