Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial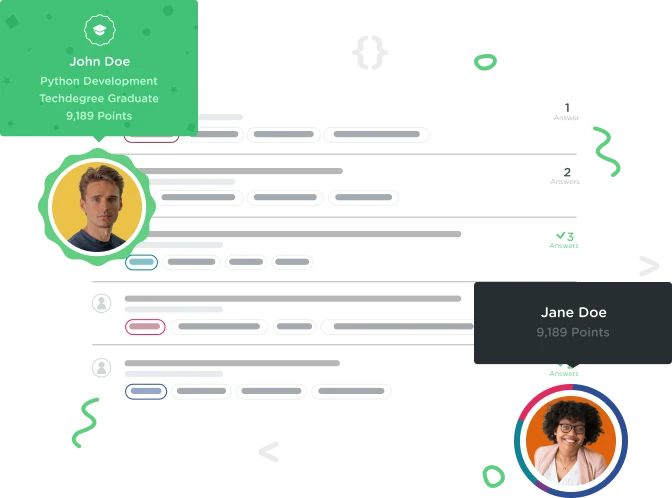
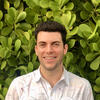
justlevy
6,325 PointsUnderstanding pytz process ... Naive, Aware, UTC, then any timezone?
I'm still wrapping my head on the process of taking a naive datetime, shifting to the correct timezone then UTC. From there we can shift to different timezones.
Is my understanding correct?
I notated Ken's code for the pytz challenge below. Hope this helps.
# Use pytz and datetime
# take date and time in MY timezone
# return in 6 different timezones using format string (fmt = '%Y-%m-%d %H:%M:%S %Z%z')
from datetime import datetime
import pytz
# Create list of different timezones which can be iterated through.
OTHER_TIMEZONES = [
pytz.timezone('US/Eastern'),
pytz.timezone('Pacific/Auckland'),
pytz.timezone('Asia/Calcutta'),
pytz.timezone('UTC'),
pytz.timezone('Europe/Paris'),
pytz.timezone('Africa/Khartoum')
]
fmt = '%Y-%m-%d %H:%M:%S %Z%z' # format datetime object
while True: # run until condition is False (break)
date_input = input("When is your meeting? Please use MM/DD/YYYY HH:MM format. ") # Ask user for specific date format and assign to date_input variable
try:
local_date = datetime.strptime(date_input, '%m/%d/%Y %H:%M') # assign local date and format in date object from a string (date_input) # why can't this take a 'fmt' variable?
except ValueError: # an invalid user inputted date format will return this error statement
print(f"{date_input} doesn't seem to be a valid date & time.")
else:
local_date = pytz.timezone('US/Pacific').localize(local_date) # transforming naive time (local_date) to aware and setting to timezone 'Pacific'
utc_date = local_date.astimezone(pytz.utc) # difficult for me to understand this step. Shifting local_date to UTC time and assigning to utc_date
output = [] # empty list to store results which will be printed
for timezone in OTHER_TIMEZONES: # loop to iterate through OTHER_TIMEZONES
output.append(utc_date.astimezone(timezone)) # take utc_date, shift to each timezone in OTHER_TIMEZONES list, add result to output list
for appointment in output: # loop for printing results stored in output
print(appointment.strftime(fmt)) # format datetime object using formating from above then print
break # stop program (end While loop)
1 Answer
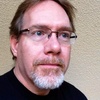
Chris Freeman
Treehouse Moderator 68,426 PointsHey justlevy , Yes, you have the gist. One big reason to go to UTC first is to work around problems with localtime. Nicely documented code.
Code feedback: flow of the code could be simplified to:
while True: # run until condition is False (break)
date_input = input("When is your meeting? Please use MM/DD/YYYY HH:MM format. ") # Ask user for specific date format and assign to date_input variable
try:
local_date = datetime.strptime(date_input, '%m/%d/%Y %H:%M') # assign local date and format in date object from a string (date_input) # why can't this take a 'fmt' variable?
break # end While loop if finished with it
except ValueError: # an invalid user inputted date format will return this error statement
print(f"{date_input} doesn't seem to be a valid date & time.")
# none of the following needs to be within the while loop or the try/except/else block
local_date = pytz.timezone('US/Pacific').localize(local_date) # transforming naive time (local_date) to aware and setting to timezone 'Pacific'
utc_date = local_date.astimezone(pytz.utc) # difficult for me to understand this step. Shifting local_date to UTC time and assigning to utc_date
output = [] # empty list to store results which will be printed
for timezone in OTHER_TIMEZONES: # loop to iterate through OTHER_TIMEZONES
output.append(utc_date.astimezone(timezone)) # take utc_date, shift to each timezone in OTHER_TIMEZONES list, add result to output list
for appointment in output: # loop for printing results stored in output
print(appointment.strftime(fmt)) # format datetime object using formating from above then print
justlevy
6,325 Pointsjustlevy
6,325 Pointsthat's 🔥 - thank you