Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial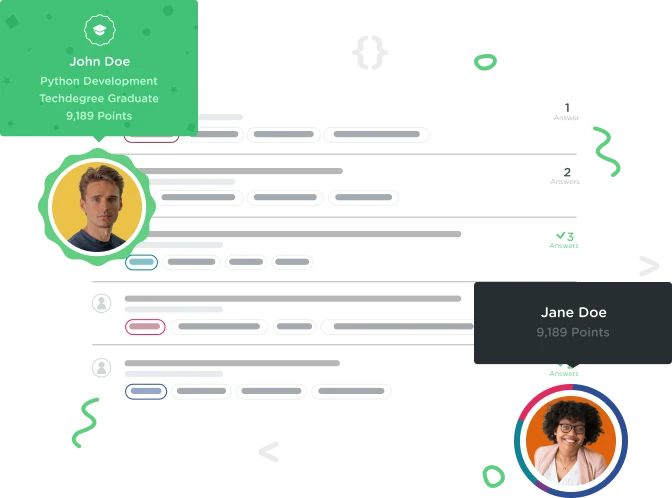
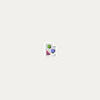
doesitmatter
12,885 PointsUnresolved attribute reference in IDE
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
setattr(self, "color", color)
setattr(self, "fuel_remaining", fuel_remaining)
setattr(self, "laps", 0)
for a, v in kwargs.items():
setattr(self, a, v)
def run_lap(self, length):
self.fuel_remaining -= (length * 0.125)
self.laps += 1
This code passes the challenge, however, when I run it in my IDE which is PyCharm, I get an unresolved attribute reference notification (not error or anything).
It recommends me to initiate the values for fuel_remaining
and laps
within the __init__
method. Like so:
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.fuel_remaining = None
self.laps = None
setattr(self, "color", color)
setattr(self, "fuel_remaining", fuel_remaining)
setattr(self, "laps", 0)
for a, v in kwargs.items():
setattr(self, a, v)
def run_lap(self, length):
self.fuel_remaining -= (length * 0.125)
self.laps += 1
I would like to know what the best practice is in this situation, should I just ignore my IDE's notification on this or is it better to initiate the values which are used inside of a class in a way other than setattr()
?
EDIT:
I think I found the answer myself. So I believe now that setattr()
should only be used if the attribute name and value are unknown. Otherwise self.name = vale
should be used. Am I correct on this?
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.fuel_remaining = fuel_remaining
self.laps = 0
self.color = color
for a, v in kwargs.items():
setattr(self, a, v)
def run_lap(self, length):
self.fuel_remaining -= (length * 0.125)
self.laps += 1
1 Answer

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsHey,
I think you're correct. Since your class already has fuel_remaining
and color
properties, I don't think you need to use setattr
to add those attributes. Essentially, I think PyCharm is saying "hey, the class has already got fuel_remaining
and color
properties, so just instantiate them directly with self.fuel_remaining = fuel_remaining
instead of using setattr
".
I hope that helps! Let me know if you have any more questions!