Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial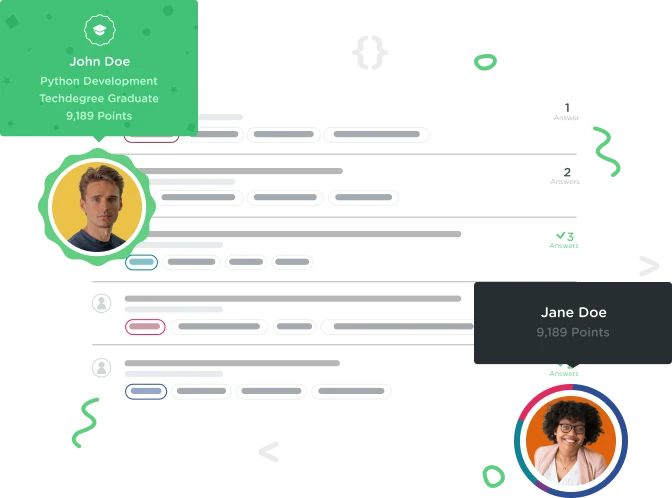
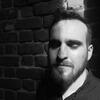
matthew mahoney
Python Development Techdegree Student 2,536 Pointsusing a function on only one part of an f string.
Hey, so I am learning about defining functions. In a little practice project I'm doing I want to only yell the user's inputted name using an f string. I tried doing this
print(f"Okay, {yell(user_name)}, well practicing our yelling function only yelling your first name!")
but it printed user_name in a separate line and the f string displayed "none" in place of the user name. I am aware that I printed twice once with my function and once with my f string print statement.
What's the propper way to use a function on only one part of an f string?
my code:
def yell(text):
text = text.upper()
num_of_chars = len(text)
print(text + "!" * (num_of_chars // 2))
yell("hello")
yell("this is a yelling function")
yell("Lets try some things out. What's your name? ")
user_name = input()
#print(f"Okay, {user_name}, we'll just practice the yelling function using your name.")
yell(f"Okay, {user_name}, we'll just practice the yelling function using your name.")
Mod edit: added markdown formatting for code readability. Check out the "Markdown Cheatsheet" linked below the comment box for examples on how to format code in your posts.
2 Answers
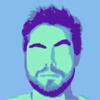
Cameron Childres
11,818 PointsHi Matthew,
If I understand correctly you're wanting to print something like this by putting your yell function inside a print statement:
- Okay, MATTHEW!!, well practicing our yelling function only yelling your first name!
What's tripping you up is that the yell function itself is also trying to print and doesn't return anything. To accomplish your goal you can replace print
with return
so that it returns the result of the function. Try this code out:
def yell(text):
text = text.upper()
num_of_chars = len(text)
return(text + "!" * (num_of_chars // 2))
print(yell("Lets try some things out. What's your name? "))
user_name = input()
print(f"Okay, {yell(user_name)}, well practicing our yelling function only yelling your first name!")
Notice that without the print statement in the function something like yell("hello")
won't print anything to the console anymore. You can wrap those lines in print() to get them to show. You can find out more about returns in the lesson All About Returns.
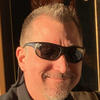
Peter Vann
36,427 PointsHi Matt!
Can you give me a better verbal description of what the yell function should accomplish?
I don't have a clear sense of its purpose.
Thanks.
-Pete
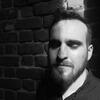
matthew mahoney
Python Development Techdegree Student 2,536 PointsHey Pete, Thanks for taking the time to answer my question. Cameron answered it perfectly. I was trying to get the yell function to only yell one part of my print statement.
Best Matt
matthew mahoney
Python Development Techdegree Student 2,536 Pointsmatthew mahoney
Python Development Techdegree Student 2,536 PointsAwesome! Thanks spooo much. This really answered my question.
P.S thanks for ignoring grammar and spelling mistakes haha.
Best, Matt