Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial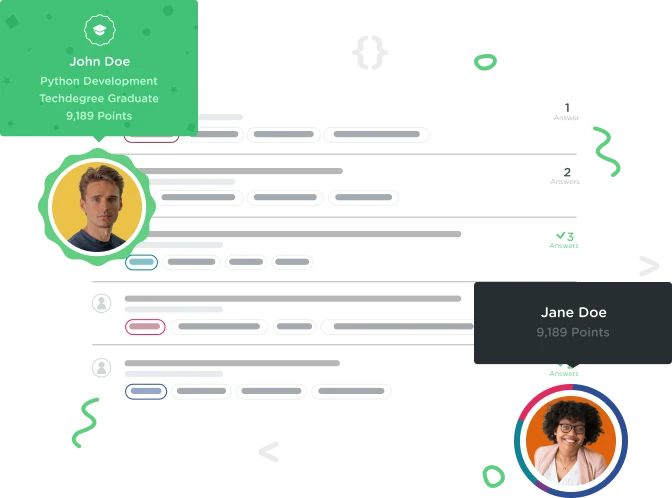

Bryce Hunter
1,186 PointsUsing the printf method, display a message that says, "First name: ", followed by the first name that the user has enter
I keep getting a message that says "Did you forget to pass the 'firstName' parameter to the printf function?" I've been stuck on this for two days. Can someone help?
// I have imported java.io.Console for you. It is a variable called console.
String firstName = ("Bryce");
console.readLine("%s, firstName");
String lastName = ("Hunter");
console.readLine("%s, lastName");
console.printf("First name: %s ,firstName");
3 Answers
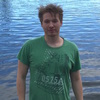
Emil Rais
26,873 PointsIt is only String-literals that are enclosed in quotes - not variables.
console.printf("First name: %s, firstName");
Should actually be:
console.printf("First name: %s", firstName);
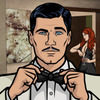
Jakob Wozniak
17,896 PointsAs Emil said, you'll want to make sure your firstName equals whatever someone enters in console.readLine:
String firstName = console.readLine("First name: ");
This means that whatever someone enters into the readLine function will be stored in firstName. This is just a shorter/condensed way of writing:
String firstName = "Bryce";
firstName = console.readLine("First name: ");
// in this code, 'Bryce' will be immediately overwritten by what the user enters in the second line ;)
I hope this helps!

Ted Barlow
6,440 Pointsthats tha way it should go, and dont forget to close the first two line with \n
// I have imported java.io.Console for you. It is a variable called console. String firstName = console.readLine("Renald\n "); String lastName = console.readLine("Toussaint\n"); console.printf("First name: %s", firstName);
Bryce Hunter
1,186 PointsBryce Hunter
1,186 PointsI'm still getting the same error message
Emil Rais
26,873 PointsEmil Rais
26,873 PointsThe output of your calls to console.readLine() should be stored in the variables. Rather than:
String firstName = "Bryce";
You should do:
String firstName = console.readLine();
Does that help you?