Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial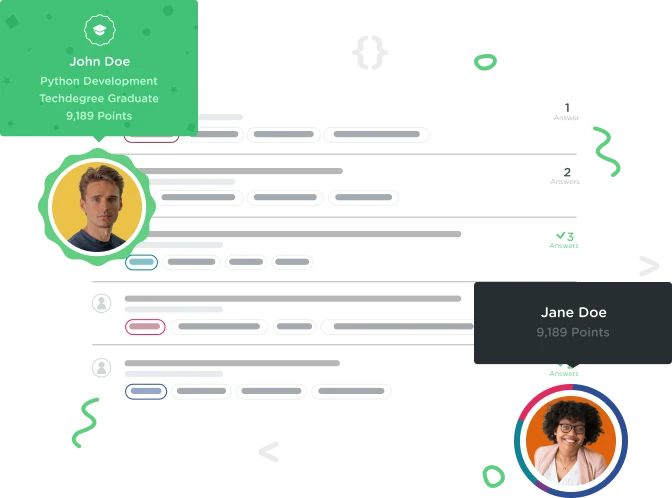

premsai kandagatla
Courses Plus Student 5,142 PointsWhat are the recommended HTTP Verbs used in Applications, and why HTTP POST is preferable than PUT and DELETE?
Why most of the Big companies use POST RESTful API calls for creating / Deleting / Updating data in database instead of PUT and DELETE.
1 Answer
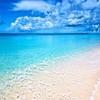
eodell
26,386 PointsHi, you can check this link to find the supported HTTP methods.
Regarding the difference between POST and PUT, it can be pretty complex but essentially it comes down to a term called idempotence. For an operation to be idempotent, it means that you can execute it multiple times expecting the same result.
So lets compare this to databases, as that seems to be the common way that the differences between the POST and PUT methods are explained.
In a database if you want to create a user in a table you could use something like:
INSERT INTO users (id, username, skill) VALUES (205, 'premsai', 'programmer');
In this table, the users.id field is the primary key and must be unique (the username would be as well but lets ignore that for this example).
So if you want to change the user's skill you could execute something similar to the following:
UPDATE users SET skill = 'developer' where id = 205;
You can execute the UPDATE statement over and over again, without any issues at all; however, you can only execute the INSERT statement once. If you tried to do the following:
INSERT INTO users (id, username, skill) VALUES (205, 'premsai', 'programmer');
INSERT INTO users (id, username, skill) VALUES (205, 'premsai', 'developer');
INSERT INTO users (id, username, skill) VALUES (205, 'premsai', 'programmer');
You would violate the USERS table's integrity constraints because you cannot insert multiple records with the ID of 205.
But you can execute:
UPDATE users SET skill = 'developer' where id = 205;
UPDATE users SET skill = 'programmer' where id = 205;
UPDATE users SET skill = 'developer' where id = 205;
As many times as you want because the UPDATE statement is considered to be idempotent. To bring this back to the HTTP requests, POST is not considered to be idempotent, meaning that if you were to execute the same statement multiple times, it would be invalid or cause an error, whereas PUT can be executed as many times as you want without issue.
That is a pretty basic explanation for a fairly complex subject. I'd highly recommend you do some more research on it and see what else you can find. The more you build/use APIs, the more you'll discover which requests are best for certain usages.
I hope this helps.
premsai kandagatla
Courses Plus Student 5,142 Pointspremsai kandagatla
Courses Plus Student 5,142 PointsIn all of my applications we are using POST methods even for INSERT statements but we are writing logic in stored procedures if id is available or not.
Due to any network latency if single users submit multiple requests or multiple users submits requests in this scenario what will be the recommended HTTP Verb to maintain the load balance of the servers?