Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial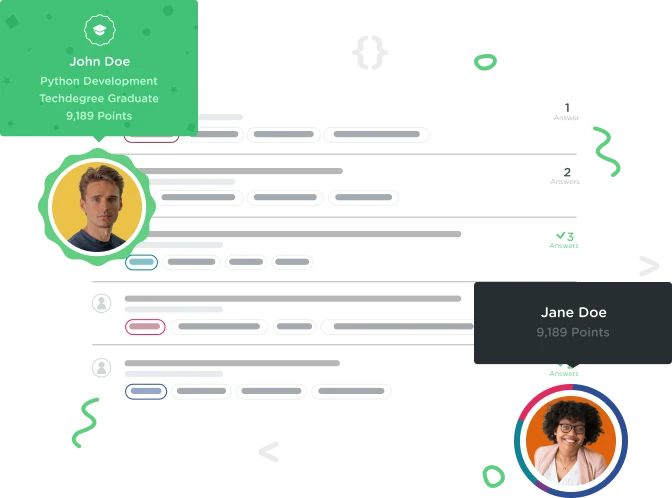

mark himself
2,048 Pointswhat do i use if not an if statement
what should i use if not an if statement to return either a true or false response
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
if (tiles.indexOf(char tile) != -1){
return false;
}
return true;
}
}
1 Answer

andren
28,558 PointsYour code is actually not quite right (even ignoring the use of the if
statement) but it's close enough that the code checker mistakenly thinks it's right.
When you pass an argument to a method (like indexOf
) you shouldn't specify the type of the argument, the type is only specified when you define a parameter or variable, so you should not include the char
keyword in your call to indexOf
.
Also the expression tiles.indexOf(tile) != -1
returns True
if the tile is present in the tiles
string and False
otherwise, so inside your if
statement you should actually return True
not False
, and vice versa for the return statement you have placed outside the if
statement.
With those two changes your code would work, but as the challenge checker hints at it's not actually necessary to use an if
statement for this challenge. As I mentioned above the tiles.indexOf(tile) != -1
expression evaluates to True
or False
on it's own, which means that you can return it directly without using an if
statement.
Like this:
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
return tiles.indexOf(tile) != -1;
}
}

mark himself
2,048 Pointsthanks. what i was having a problem with was how to answer the question of the prompt. i didn't know that i could change 'return false' to anything else. I thought that i had to put in correct code before it got to the return part. what I needed was the piece: ""return tiles.indexOf(tile) != -1;"" . Thanks so much for your help. it wasn't a coding question, but a how do you want my answer question.
mark himself
2,048 Pointsmark himself
2,048 Pointsthis isn't necessarily wrong according to the 'check my work' answer, but it's not letting me pass through this assignment until i put what they want. i don't know what they want