Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial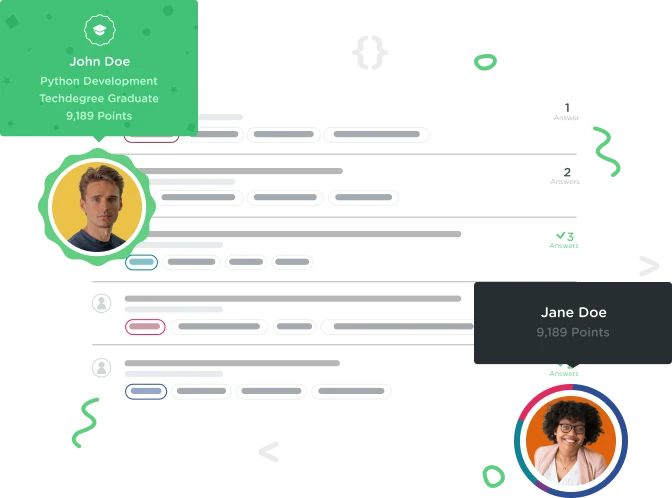

Angelus Miculek
3,859 Pointswhat does return do?
Not sure I'm getting this straight, but the purpose of the return is so the variable can store it? The variable would be empty if that value wasn't returned? Could someone explain this, thanks.
2 Answers
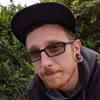
Travis Alstrand
Data Analysis Techdegree Graduate 49,443 PointsYes Angelus Miculek , you're on the right track!
In Python (and in many other programming languages), the purpose of the return
statement is to send a value back to the part of the program that called the function. If a function doesn't return a value, it doesn't send any output back, and the variable calling it would be empty (or None
, which is Python's equivalent of "no value").
Hereβs an example:
def add_numbers(a, b):
return a + b # Returns the sum of a and b
result = add_numbers(3, 4) # result will store the value 7
print(result) # This will print 7
In this case, the function add_numbers
returns the result of a + b
. The variable result
stores this returned value. If there were no return statement, result would not store anything useful:
def add_numbers(a, b):
a + b # No return statement
result = add_numbers(3, 4) # result will be None
print(result) # This will print None
Without return
, the function still performs the operation a + b
, but it doesnβt send the result back, so the calling code doesn't have access to it. That's why the return
statement is crucial when you want a function to pass a result back to the part of the code that called it.
I hope that helps it make sense π

Angelus Miculek
3,859 PointsYes very good, thanks!