Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial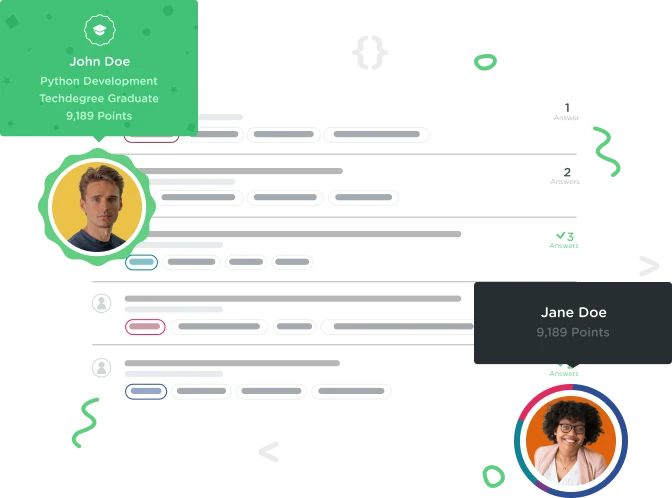

Kyree Lewis
901 PointsWhat is a variable?
What us a variable?
2 Answers
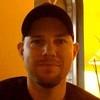
Jeremy Hill
29,567 PointsA variable is basically a placeholder for data. In java an integer or int holds whole numbers only, negative and positive numbers. The variable "double" holds decimal numbers, and the variable "String" holds letters, words, phrases, etc.
So when I say: int num1 = 5; I am saying that I am storing the number five in an int variable named "num1". I could have named it anything (with some limitations). If I do this:
int num1 = 5;
int num2 = 3;
int total = num1 + num2;
I am saying that I am storing the number five in variable num1 and storing three in the variable num2; and I am setting the variable "total" equal to the sum of variable "num1" and "num2".
I can also store my name like this:
String myName = "Jeremy"; In java, when you first declare a variable you must give it a type (String, int, double, float, long, etc). Once the variable has been declared you no longer have to add the variable type when you reference the variable; like this: int num1 = 5; int num2 = 3;
num1 = 8;
int total = num1 + num2; //total would now equal 11
I hope this helps.

Simon Coates
28,695 PointsA variable is a named placeholder for a value. Some tutorials use the analogy of a box/bucket, where you can put something new in the bucket, or ask what is in the bucket at a moment of time. In java, you specify the type of the variable - which limits what can be stored in it. (at a more advanced level there are considerations about where/how long the variable is able to be access on that name. this is called scope.)