Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial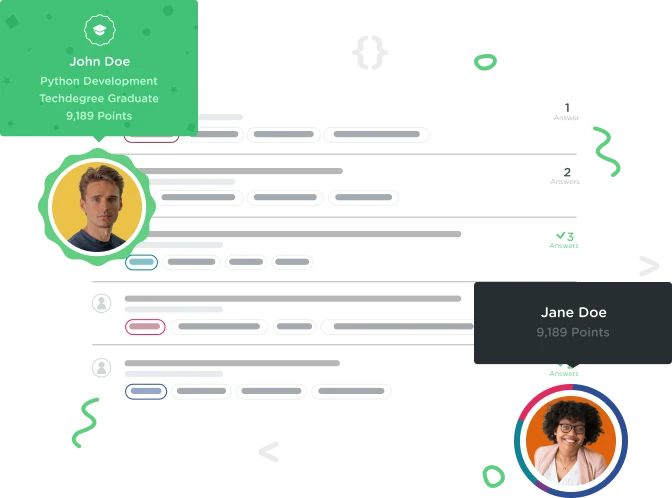
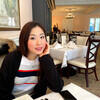
Hanwen Zhang
20,084 PointsWhat is the difference between class and function in Object-Oriented Programming?
Any major concepts for each one?
1 Answer
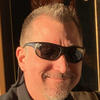
Peter Vann
36,427 PointsHi Hanwen!
In very simple terms, functions are essentially just small blocks of code that you can encapsulate and name, therefore making that code easily reusable. Sometimes they are referred to as procedures or subroutines.
Example of a function:
function square(num) {
return num * num;
}
console.log( square(5) ); // logs 25
Classes, on the other hand, are more like blueprints for objects. Objects are specific instances of classes that can have properties (which store information about the object and possible pieces of "state") and methods, which are functions within the class, that can manipulate, validate, present, etc. that objects properties. Classes/objects often emulate real-world objects, such as cars or bank accounts.
Example of a class:
class Car {
constructor(name, year, gas) {
this.name = name;
this.year = year;
this.gas = gas
this.odometer = 0; // default - new car has 0 miles
}
drive(miles) {
this.odometer = this.odometer + miles;
this.gas = this.gas - (miles/20); // 20 miles to the gallon
}
}
let myCar = new Car("VW", "1972", 20);
myCar.drive(40); // Drive 40 miles & use up 2 gallons of gas
console.log(myCar.gas); // now 18 gallons
console.log(myCar.odometer); // now reads 40 miles
More info
Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedureβa set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. To use a function, you must define it somewhere in the scope from which you wish to call it.
From here:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Functions
Also:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes/constructor
JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects, properties, functions, and methods, and how to create your own objects.
From here:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Working_with_Objects
I hope that helps.
Stay safe and happy coding!