Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial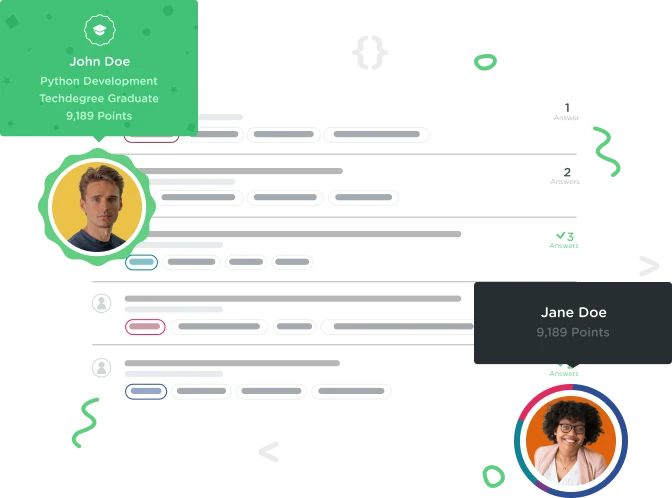

JASON LEE
17,352 PointsWhat is the difference between class attributes and instance attributes?
In Python, what is the difference between class attributes and instance attributes? The only difference I can tell is that the instance attributes can be initialized when creating a new Class variable via the arguments passed to it. Otherwise, the behavior seems the same.
For instance, the self.is_moving = False
property is not being passed as an argument in def__init__(self ....)
, so it can be moved up to the class attribute section as .is_moving = False
and it seems to get the same result. Same with self.gas = 100
class Car:
# class attributes, NOT TO Be confused with class INSTANCE
wheels = 4
doors = 2
engine = True
def __init__(self, model, year, make="Ford"): # These are instance attributes created with an initializer inside the class. Initializer is a method called __init__ | #best practice to put the default arguments at the end
self.make = make
self.model = model
self.year = year
self.gas = 100
self.is_moving = False
def stop(self):
if self.is_moving:
print('The car has stopped')
self.is_moving = False
else:
print('But the car ain\'t moving..')
def go(self, speed):
if self.use_gas():
if not self.is_moving:
print(f'The car starts moving ...')
self.is_moving = True
print(f'The car is going {speed}')
else:
print('You have run out of gas')
self.stop()
def use_gas(self):
self.gas -= 50
if self.gas <= 0:
return False
else:
return True
```
1 Answer
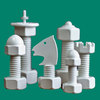
Steven Parker
231,007 PointsThe main difference is that a class attribute is shared by all instances of that class, and an instance attribute is unique to each instance. So if you change a class attribute in one instance, it will also change for every other instance.
So it makes sense for "wheels" to be a class attribute, since all cars have 4 of them and it won't change. But the amount of gas would be different for each car, so that makes more sense as an instance attribute.