Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial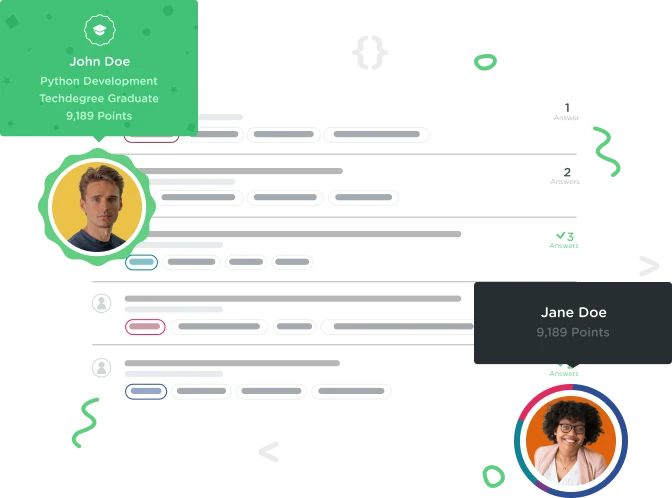

Loren Cardella
Python Web Development Techdegree Student 8,096 PointsWhat is 'try' in Python?
I completed the Python Basics course without understanding how 'try' and 'except' work. I always got errors from them. I only know how to use 'if' to ask for the input, 'elif' to cover each possible incorrect input and 'else' for the correct input. An instance of 'except' in the course looks like an incomplete 'elif' list to me. And I don't see how 'ValueError' and 'TypeError' get the user back to being able to enter the input over again.
4 Answers
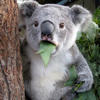
ursaminor
11,271 PointsYou code will never execute what's in the except clause because the code in the try clause will run with no problem, even if the user doesn't enter a name. To make it raise an error, you have to use the keyword raise
. Then the code in the except clause (with the same exception class) will run. There, you can make it keep asking the user for their name until they give it. Then, the program will exit the except clause and go on to print the statement.
try:
name = input("What is your name? ")
if name == '':
raise ValueError # force an error
except ValueError:
while not name:
name = input("Name cannot be blank. What is your name? ")
print("Your name is ", name, ".", sep="")
This is kind of a contrived example, because you could do the same thing without the try and except:
name = input("What is your name? ")
while not name:
name = input("Name can't be blank. What is your name? ")
print("Your name is ", name, ".", sep="")
By the way here's a better way to do the print statement: print("Your name is {}.".format(name))
Here's an example where the code itself could raise an exception, so you're not raising your own:
try:
age = int(input("How old are you? "))
except ValueError:
age = input("It doesn't look like you entered a number. Please enter a number.")
If the user enters "sixteen" or something that can't be turned into an int
, a ValueError will be raised and so the code in the except clause will run.
Here's more info on exception handling: https://docs.python.org/3/tutorial/errors.html
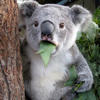
ursaminor
11,271 Pointstry and except are used when there could be a runtime error -- an error that happens during execution of the program. For example, say a program opens a file and read/write to it, and the file is corrupt or can't be found for some reason. If there was no error handling it would just crash the program and output a bunch of stack trace that's gobbledygook to most people. So instead you would put in some helpful message (or ask the user for another input in the example you're talking about) in the except block so it doesn't crash the program. With if statements, you do something if some condition is true. With try and except, you try something and if it doesn't work (because of something that happens during execution, that you can't necessarily control) then you handle the problem. It's kind of like asking for permission vs asking for forgiveness.

Loren Cardella
Python Web Development Techdegree Student 8,096 PointsCan you elaborate on how 'except', 'ValueError' and 'TypeError' give the user another chance to enter a valid input?
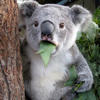
ursaminor
11,271 PointsCan you paste in the code? It's been a long time since I took the course.

Loren Cardella
Python Web Development Techdegree Student 8,096 PointsHere's a basic attempt at using 'try' and 'except':
try:
name = input("What is your name? ")
print("Your name is ", name, ".", sep="")
except name == "":
raise ValueError("Please type your name.")

Prashant Nayak
2,300 Pointstry & except is used to make the code more robust and not fail abruptly because of error.
example: Our program is adding 2 number entered by the user. Program expect both the entries to be an integer but if the user enters string, program will through error.
try: age = input("What is your age? ") print ("Your age is: ", int(age)) except ValueError: print('please enter your age as nunber')
Loren Cardella
Python Web Development Techdegree Student 8,096 PointsLoren Cardella
Python Web Development Techdegree Student 8,096 PointsI appreciate the effort you clearly invested to help me. Unfortunately, I still don't get it. I'll just revisit the issue some other time.