Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial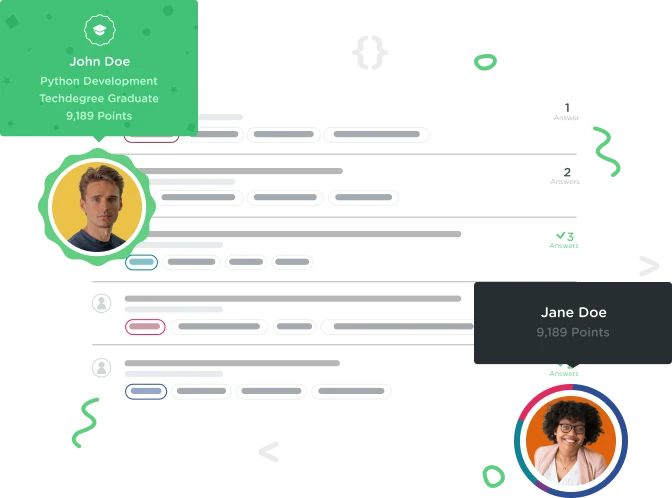

Abhilasha jangid
2,925 Pointswhat the type of normalizeDiscountCode method & why
how take the discount code in the method.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private normalizeDiscountCode(){
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = discountCode.isUpperCase();
}
}
1 Answer
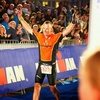
Steve Hunter
57,712 PointsHi there,
The first part of this challenge has two steps.
First, create the new private
method called normalizeDiscountCode()
. This returns a String
and takes one parameter, which is also a String
.
You've got:
private normalizeDiscountCode(){
}
OK - so we need to add a return data type (String
) so we get:
private String normalizeDiscountCode(){
}
And then we need a parameter - I'll call it discountCode
since that's what it is:
private String normalizeDiscountCode(String discountCode){
}
So that's the method skeleton set out right. We now just need to have it actually return a string. The challenge tells us that we should return the discountCode
in upper case. For that, we use a method called toUpperCase()
, as we've seen in the videos. We have the discountCode
(we passed it into the method, right?) so we just need to chain that method on to that, using dot notation, and return it.
private String normalizeDiscountCode(String discountCode){
return discountCode.toUpperCase();
}
Sorted; that's step 1 done.
Now, we need to use this new method in the applyDiscountCode()
method. At the moment, that looks like this:
public void applyDiscountCode(String discountCode) {
this.discountCode = discountCode;
}
So it is receiving a discount code and assigning that to the member variable of the class. We want to assign the returned string from normalizeDiscountCode
into that. So, we call the method we just wrote and pass in the discountCode
. What comes back will be the upper case version (for now - there's more later) which we want to assign into this.discountCode
in the same way as now.
That look like:
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
That should get you through task one.
Let me know how you get on.
Steve.