Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial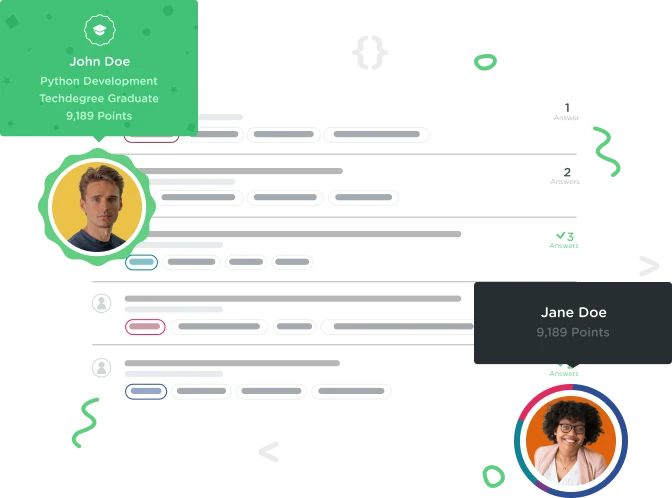
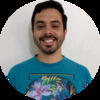
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsWhen I enter 'a', it does nothing. It just goes back to the loop again. Why?
Am I missing something?
(please disregard the comments on the code. It's in portuguesese)
#!/usr/local/pyenv/shims/python3
# Jeito ideal de organizar seus imports:
# As coisas que vem de python estão aqui
from collections import OrderedDict
import datetime
import sys
# As coisas que vem de uma third-party library
from peewee import *
db = SqliteDatabase('diary.db')
class Entry(Model):
# Declarar o field para o texto
content = TextField()
# Declarar o field para a hora e atribuir como valor default o datetime.datetime.now
timestamp = DateTimeField(datetime.datetime.now)
class Meta:
database = db
def initialize():
"""Create the database and the table if they don't exist"""
db.connect()
db.create_tables([Entry], safe=True)
def menu_loop():
"""Show the menu"""
# Apenas uma maneira de setar uma variável sem dar um valor a ela.
choice = None
while choice != 'q':
print("Enter 'q' to quit.")
for key, value in menu.items():
print('{}) {} '.format(key, value.__doc__))
choice = input('Action: ').lower().strip()
if choice in menu:
menu[choice]()
# Vamos fazer algum crud. Vamos colocá-los em funções
def add_entry():
"""Add an entry"""
print('Enter your entry. Press ctrl+d when finished.')
# sys.stdin provavelmente é um file object. Lê-se o conteúdo dele e depois o stripa.
data = sys.stdin.read().strip()
# Se o usuário tiver digitado algo
if data:
# Se o usuário entrar com qualquer valor que não seja 'n'
if input('Do you wanna save it? [Yn]').lower() != 'n':
# Crie uma model instance cujo content recebe o conteúdo da variável data.
Entry.create(content=data)
print('Your content was saved successfully!')
def view_entries():
"""View previous entries"""
def delete_entry(entry):
"""Delete an entry"""
# Lembra da ordem a qual as coisas foram adicionadas. O jeito que faz isso é porque colocamos as coisas
# como uma lista. Cada item é uma tuple, que é uma maneira bem comum de criar dictionaries.
menu = OrderedDict([
('a', add_entry),
('v', view_entries)
])
if __name__ == "__main__":
# Desse modo sabemos que a database existe antes rodemos o app:
initialize()
menu_loop()
1 Answer
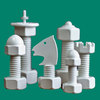
Steven Parker
240,449 PointsBased on the indentation, the loop ends right after the choice is input, and the loop continues to repeat until a choice of "q" is made.
You probably meant to code the "if" statement and the code it controls as part of the loop instead of coming after it.
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsEwerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsThank you!!!